Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial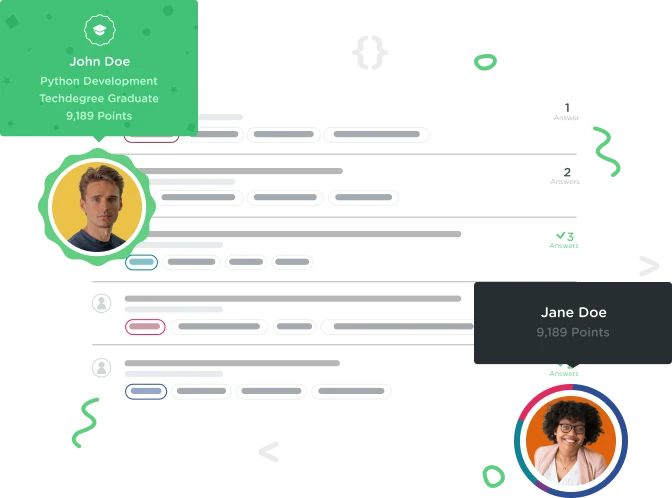

Alvin McNair
3,398 PointsShould I be expecting a list of is there some variable argument syntax I missed in one of the lessons
I'm not sure where the syntax error I'm getting is coming from
# If you need help, look up datetime.datetime.fromtimestamp()
# Also, remember that you *will not* know how many timestamps
# are coming in.
timestamp_oldest(timestamps):
timestamps.sort()
return datetime.datetime.fromtimestamp(timestamps[0])
2 Answers
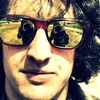
Adam Van Antwerp
3,104 PointsOkay a few things you're a bit off on here. First of all, you need the def keyword when you define your function. That's easy to miss (it took me a while to see it!) Also, your function needs to be able to receive any number of arguments (as requested in the challenge). As it stands, you can only receive one (timestamps) and I'm assuming you thought you'd be getting a list. We can use the *args concept to receive any number of arguments into a tuple (we traditionally call this args, so I will go with that). This, of course, is a tuple and doesn't have the sort command. Luckily, it's easy to convert it with a type conversion and do the sorting afterwards. This is what I came up with based on your original work.
def timestamp_oldest(*args):
timestamps = list(args)
timestamps.sort()
return datetime.datetime.fromtimestamp(timestamps[0])
I also took the liberty of indenting to 4 spaces as per PEP8 (hope you don't mind!) XD
William Li
Courses Plus Student 26,868 Pointsumm ... I wonder why the solutions for this challenge would work without importing the datetime
module first.
PM. Kenneth Love
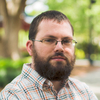
Kenneth Love
Treehouse Guest TeacherBecause I was importing datetime
in my validation code which puts it into locals()
...yeah. Removed that so now it'll require the import.
Adam Van Antwerp
3,104 PointsAdam Van Antwerp
3,104 PointsBonus: Tuples are immutable, so that's why we can't sort them. The way I outlined of explicitly converting to a list, then sorting is OK, but there's also a built in method for that, sorted()! It will convert a tuple to a list, sort it, and return that, so you could cut down on a line if you want. It would look something like
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 PointsHi, Adam Van Antwerp , I don't know about Alvin, but I really like this great answer :)