Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial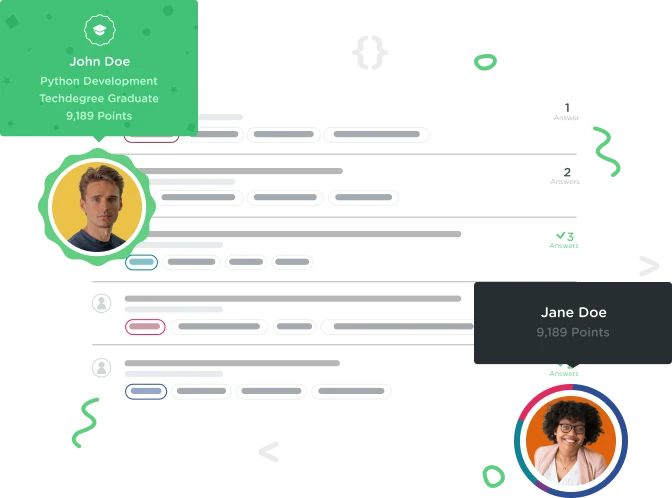
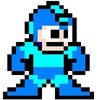
Robert Richey
Courses Plus Student 16,352 PointsShow: Python Quiz
After the planning stages for setting up the python quiz - from the Dates and Times course, I went ahead and finished it on my own. Here is a GitHub link to my Lumberjack Quiz. Feedback, criticism and praise are all welcome!
Once both files (quiz.py and questions.py) are downloaded, the quiz can run from the command line with python quiz.py
from within the same directory.
This was a fun project; looking forward to extending the dungeon game as well. Thanks Kenneth Love
Cheers
1 Answer

David Clausen
11,403 PointsLooks nice. My only suggestion...and its just a suggestion is the try/except should only focus on the possible error, you should move past the block once your try is successful...example:
while True:
#Get the input
answer = input("[Q"+str(idx)+"] "+question.text)
#Test the input
try:
answer = int(answer)
#intercept non-intergers
except:
if answer.lower() == "q" or answer.lower == "quit":
sys.exit()
print("Please only enter numbers for the answer.")
print("Or enter Q or QUIT to quit program.\n")
#Continue like break but just starts you at the loop again
#instead of terminating the loop
continue
#Now we know answer is an int or the loop would have started over
if answer == question.answer:
self.answers.append(True)
print("*** Correct! ***\n")
else:
self.answers.append(False)
print("*** Incorrect ***\n")
break
The reason why is in this code negligible but you really only want to do what is absolutely necessary in the try/except cause everything in the "try" block will excute significantly slower as to ensure it catches an error before it executes it. If you really wanna get tight and slim on the try/except for your program then"
def isNumber(inputToCheck):
try:
return int(inputToCheck)
except:
return False
Great thing about Python is its dynamic, you can return anything, here the try and except are only used to get an answer so imagine your block now:
while True:
#Get the input
answer = input("[Q"+str(idx)+"] "+question.text)
#Use our new function to convert it
answer = isNumber(answer)
#if answer was unsuccessfully converted to int it will be False
# 0 also equals false BTW
if(answer == False):
if answer.lower() == "q" or answer.lower == "quit":
sys.exit()
else:
print("Please only enter numbers for the answer.")
print("Or enter Q or QUIT to quit program.\n")
#the answer was successful so we proceed with checking if incorrect or not
else:
if answer == question.answer:
self.answers.append(True)
print("*** Correct! ***\n")
else:
self.answers.append(False)
print("*** Incorrect ***\n")
break
Again this is only important to avoid writing too much code in a try: block.
Hope this helps! Either way i ran your program and it looks nice and readable!
EDIT: Also all code here was tested so it should work, i used Python 2.7 but everything is used works in 3.
David Clausen
11,403 PointsDavid Clausen
11,403 PointsZero also equals False so my last examples has pitfalls if a question accept requires 0 as the anwser. But i wanted to make it straight forward. What I do for my isNumber function is take a page from Javascript and in my function:
I'd return NaN which mean Not A Number, and we can check that which when i check for it can't confuse zero and false
This way I can have a 0 for an answer without messing it up
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsHi David,
Thank you so much for your time and effort into this reply. I understand and agree with trimming the try / catch down to the parts that can fail; I suppose I felt a bit rushed in my excitement to 'ship it'. I do really like your solution to implement a
isNumber()
function.I'll take to heart what you've said. Thanks again, I really do appreciate the well thought-out response.
-Robert
David Clausen
11,403 PointsDavid Clausen
11,403 PointsAlso i made a comment that i hope you saw to extend my isNumber for your game as their was a logic error in the function, i forgot python considers 0 == False, the comment modifies it to something you'll be familiar with and won't run into a problem if the answer to a question is 0.
Cheers! Can't wait to see what you come up with next!