Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial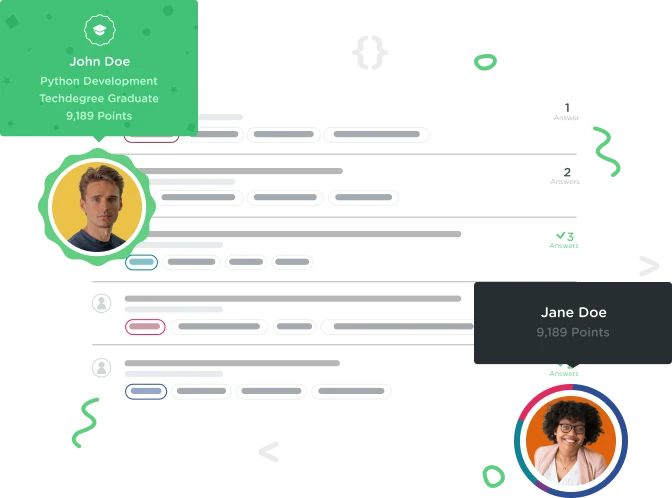

Jesse Krieg
583 PointsSillycase code: rounding seems wonky
working on the code challenge and I'm running into this issue:
def sillycase(xf):
first = round(len(xf)/2)
second = int(len(xf)/2)
return xf[:first].lower() + xf[second:].upper()
my code above works for treehouse (9 letters) but I keep getting an error when it auto tries to use 'kenneth'. In the terminal it rounds 4.5 down (treehouse) to 4, BUT it rounds 3.5 up (kenneth) up to 4. Is there a reason why it does this?
I assumed we wanted it to round down as the instructions state it should say 'treeHOUSE' not 'treehOUSE'
very new to this and assuming I'm attacking this question from the wrong direction.
Thanks
4 Answers
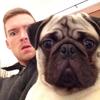
Brian Douglas
822 PointsYou could define a variable for rounding half of the original input with a float '2.0'.
I have adapted you code below and this should pass.
def sillycase(xf):
length = len(xf)
half = int(round(length / 2.0))
first = xf[:half].lower()
second = xf[half:].upper()
return first + second
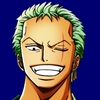
David Sudermann
Courses Plus Student 3,228 PointsPython3 is using "round towards even" as default. You could use integer-division as an alternative here:
7 // 2 = 3
7.9 // 2 = 3
So to solve this exercise you'd use:
def sillycase(xf):
first = len(xf) // 2
second = len(xf) // 2
return xf[:first].lower() + xf[second:].upper()

Dave Huckle
7,030 PointsActually you can shorten it even further
def sillycase(xf):
middle = len(xf) // 2
return xf[:middle].lower() + xf[middle:].upper()
but round() should be used: https://teamtreehouse.com/community/sillycase-code-challenge-assume-the-string-is-of-even-length
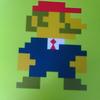
KAZUYA NAKAJIMA
8,851 PointsI can't explain well, hope this helps. http://stackoverflow.com/questions/10825926/python-3-x-rounding-behavior

fahad lashari
7,693 PointsI didn't read the comments and never saw the comment about using round(). I just hacked together a simply solution and it worked. Hope this helps:
def sillycase(word):
string = word[:4].lower()
string+=word[4:8].upper()
return string
sillycase("Treehouse")