Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial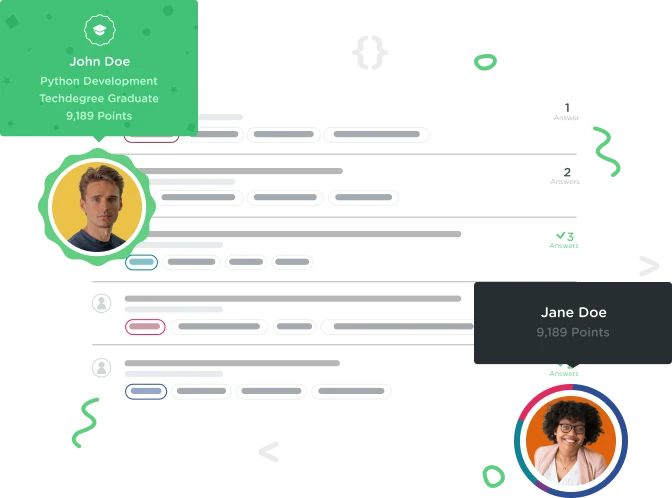

Benjamin Bradshaw
3,208 PointsSome kinda strangeness is happening.
I'm on task 5 but somehow, whenever I submit my answer it says that task 4 is no longer working. When I go back to task 4 it works just fine. My question is, why is this happening and how does my task 5 look?
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
teachers = {'Benj': ['Omnisciences'], 'Heather': ['Social Therory'] , 'Chris': ['Game Therory', 'Food Studies'], 'Abe': ['studystudies', 'allstudies']}
def num_teachers(teachers):
count = 0
for key in teachers:
count += 1
return count
def num_courses(teachers):
num = 0
for courses in teachers.values():
num += len(courses)
return num
def courses(teachers):
new_list = []
for value in teachers.values():
new_list += value
return new_list
def most_courses(teachers):
max_count = 0
most_courses = str()
for teacher, course in teachers.items():
if len(course) > max_count:
max_count += len(course)
most_courses = teacher
return most_courses
def stats(teachers):
stat_list = []
for item in teachers:
current_teacher = []
current_teacher = [item, len(teachers[item])]
stat_list.append(current_teacher)
return stat_list
1 Answer
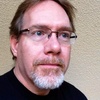
Chris Freeman
Treehouse Moderator 68,423 PointsWhen a previously passing task now fails, it usually means that a new syntax error was introduced while working on the current task. In this case, it looks like the return
statement for the previous most_courses
function has gotten indented inside the for
loop.
Also it looks like the max_count
is using a += instead of a simple assignment.
Benjamin Bradshaw
3,208 PointsBenjamin Bradshaw
3,208 PointsThe syntax was the problem. It worked with the += so I'm wondering why I would want to use an assignment instead. should it not have worked? Is there and advantage to using an assignment over a += ?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsRevisiting this question got me thinking....
The fact your code passes with the "+= in place is a lucky coincidence due to the order the teachers are retrieved from the
teachers
dict test code. Running the code with slightly differing data yields wrong results. I added print message to your code to watch for a failing case. Below is a example of a failing case.You can see from the above example using += has undesired behavior. Using = is the correct solution.
Tagging Kenneth Love to review the challenge test data to be sure to catch the misuse of +=.