Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial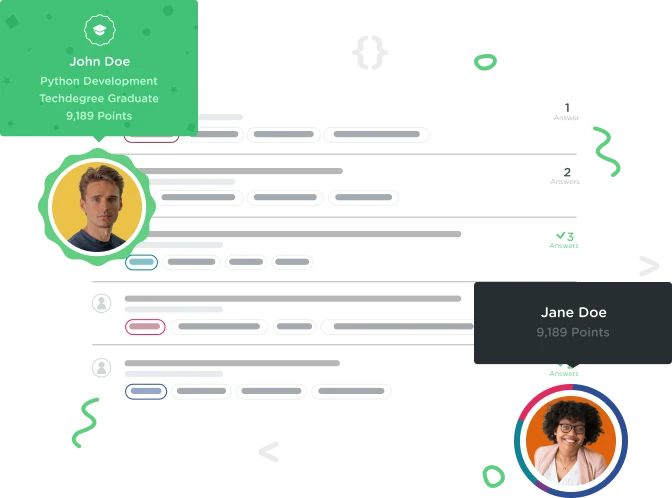
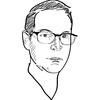
Timothy Comeau
3,710 PointsStuck on Challenge of Python functions
Ok, now I'm stuck on question 2.
This code works outside of the Challenge interpreter to deliver the requested result.
Question 1 is: Make a function named add_list that adds all of the items in a passed-in list together and returns the total. Assume the list contains only numbers. You'll probably want to use a for loop.
and it's accepted answer is:
list=[1,2,3]
def add_list(list):
sum = 0
for num in list:
sum = sum + num
return sum
b= add_list(list)
print(b)
Question 2 is: Now, make a function named summarize that also takes a list. It should return the string "The sum of X is Y.", replacing "X" with the string version of the list and "Y" with the sum total of the list.
list=[1,2,3]
def add_list(list):
sum = 0
for num in list:
sum = sum + num
return sum
b= add_list(list)
print(b)
#Q2 answer
def summarize(list,b):
a=str(list)
print("The sum of {} is {}".format(a,b))
c = summarize(list,b)
and this outputs
The sum of [1,2,3] is 6
but the challenge interpreter throws a "summarize() missing 1 required positional argument: 'b'" error and if I modify it I get a "no longer passing error"
6 Answers
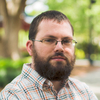
Kenneth Love
Treehouse Guest TeacherYou've said that summarize
takes two arguments, list
and b
. When I call summarize
to check your work, I only pass in one argument, the list I want summarized. That's why you're getting that error.
On a side note, you don't want to name a variable list
(or str
or dict
or...you get the idea) as that'll make it hard for you to do list()
to turn something, say a string, into a list.
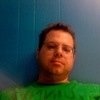
Blake Hutchinson
21,695 PointsThis worked for me.
def summarize(list):
return "The sum of {} is {}.".format(str(list), sum(list))
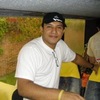
Walter Cordero
10,850 Pointsin this challenge, in task 2, I've wrote this code:
list=[1,2,3]
def add_list(list): sum = 0 for num in list: sum = sum + num return sum
def summarize(): return "The sum of {} is {}".format(str(list).strip('[]'), add_list(list))
sum = summarize()
print (sum)
my output is: The sum of 1, 2, 3 is 6
and I get this error message: "Oops! It looks like Task 1 is no longer passing."
Where or which is my error? thanks.

alec burnett
2,501 Pointsi tried this and tried every thing on google etc. i need help please
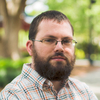
Kenneth Love
Treehouse Guest TeacherWe can't really help you without seeing your code.

alec burnett
2,501 Pointswell how do i show it kenneth love
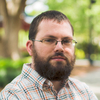
Kenneth Love
Treehouse Guest TeacherCopy it from the code challenge and paste it into a comment/answer box on this thread or start a new thread with it. Put three backticks (```) and the word "python" before the code, and three more backticks at the end of it. It'll be something like:
```python
def hello():
print("hello")
` ` `
but without the spaces in the last set of backticks.
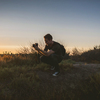
Shea Taylor
Courses Plus Student 664 PointsI know this is an old question... but I had some trouble with it myself and thought I'd revive it. Why not solve with the sum function? Like so:
my_list = [1, 2, 3]
total = sum(my_list)
def add_list(my_list):
return total
print(add_list(my_list))
def summarize(my_list):
return "The sum of {} is {}.".format(str(my_list), total)
print(summarize(my_list))
This is what ultimately worked for me. Thoughts? What would be the benefit of a for loop in this situation?
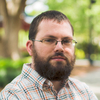
Kenneth Love
Treehouse Guest TeacherYour solution assumes that my_list
and total
would be available. Think about if you were writing this as a standalone function where the function's arguments would be coming from somewhere else, not sitting in the same file.
Also, the point of the exercise is to build your own version of sum
, effectively.
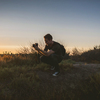
Shea Taylor
Courses Plus Student 664 PointsKenneth Love I appreciate the response! I'm pretty new to coding, so forgive my ignorance. I see what you're saying, but how would I code this function without referencing my_list
? I know how to build my own version of sum
into the function, but I'm not sure how to code it to take an argument outside of the file. What do we put in the parentheses if we wanted to make it standalone?
def add_list(???):
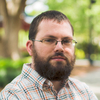
Kenneth Love
Treehouse Guest TeacherShea Taylor You put what you want the argument to be called inside of the function.
def add_list(list_to_add):
def add_list(bucket_list):
def add_list(stuff):
Whatever you call the argument is what its value will be known as inside of the function.
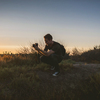
Shea Taylor
Courses Plus Student 664 PointsKenneth Love Got it! Thank you for the clarification.
Timothy Comeau
3,710 PointsTimothy Comeau
3,710 PointsOk, noted about the bad name for the list variable.
So I'm trying to call variable b because it contains the function add_list and its output/result. So I'm thinking that to run summarize, I need to pass in list (to turn it to a string) and add_list - but can I pass in a function as an argument to another function? I.E. ...
def summarize (list, add_list()):
??
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherDon't try to call functions as arguments when creating functions because that won't work.
But you can call functions as arguments to other functions or inside of functions.
Are both valid.
Timothy Comeau
3,710 PointsTimothy Comeau
3,710 PointsSorry clicking on "add a comment" turned that into "best answer"
I don't understand what you're saying above, since it seems to be contradictory. You're saying 1) Don't call functions as arguments when creating functions, but 2) call functions as arguments. ???? What is the difference.
I'm running solutions ideas on my own Terminal and default Mac Python and like above, I found this script will also return the expected result. but when I run it in the code interpreter, I once again get a "no longer passing error";
But I'm also getting the None error when I run the script
Kenneth Love
Treehouse Guest TeacherKenneth Love
Treehouse Guest TeacherLet's see if this helps explain it a bit better.
Defining a function
I've created a function using the
def
(short for "define") keyword. I've said the function takes two arguments.Calling a function
my_function(str(100), str(200))
Here I've called the function named
my_function
and given it the two arguments it expects. Each of my arguments to it, though, are actually calling thestr()
function, too.So you can call functions as arguments to another function, but you can't call a function when you're defining a function.
Also, your
summarize()
function doesn't take any arguments, so it doesn't get a list to summarize, it always works on theex_list
variable that you create at the top. That won't pass the test and wouldn't be a very useful function in the real world.