Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial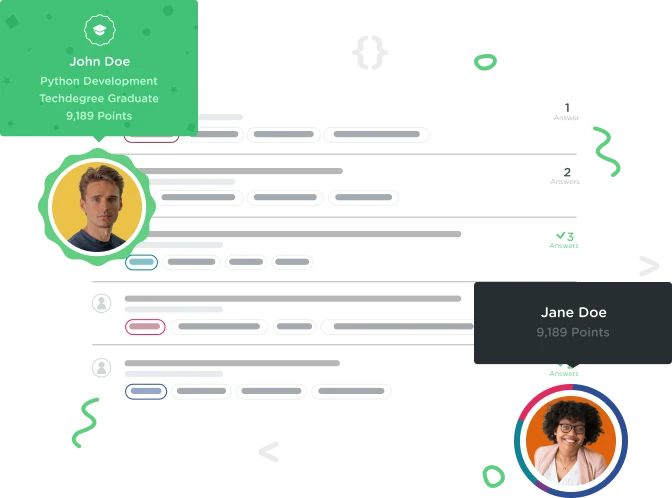

Ralph Nicholson
812 PointsSwift 2.0 Collections and Control Flow - While Loops
Now that we have the while loop set up, it's time to compute the sum! Using the value of counter as an index value, retrieve each value from the array and add it to the value of sum.
For example: sum = sum + newValue. Or you could use the compound addition operator sum += newValue where newValue is the value retrieved from the array.
Completed first task and I am stuck on the second part of this challenge task, asking to add the value of sum to a new value
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
print(numbers[counter])
counter++
}
while counter < numbers.count {
var newValue = numbers[counter]
sum += newValue
counter++
}
3 Answers
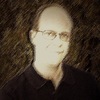
Jason Anders
Treehouse Moderator 145,860 PointsHey Ralph,
I think you may be overthinking this one a bit. The second part of the challenge is pretty much like the first, except with a variable sum
instead of a print
statement. So, you only need to add one line of code to the while loop
you wrote for Task 1.
The challenge task question was just using newValue
as an example. But, what you need is to add all the numbers being pulled from the array to each other. You are already printing each number, so in the same way you are getting those numbers, we can add them to each other with sum += numbers[counter]
(just like the print).
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
print(numbers[counter])
sum += numbers[counter]
counter++
}
Hope that helps
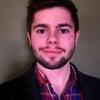
Paul Brazell
14,371 PointsYou don't need two while loops. Just change your print statement from the first while loop to the addition. You also don't need to assign the numbers[counter] to a new variable. Easy as this...
let numbers = [2,8,1,16,4,3,9]
var sum = 0
var counter = 0
// Enter your code below
while counter < numbers.count {
sum += numbers[counter]
counter++
}

Ralph Nicholson
812 PointsThanks Paul, appreciate it!

irfan Rabbani
656 Pointskeep things simple and i would suggest do it like: while counter < numbers.count { counter += 1}
Paul Brazell
14,371 PointsPaul Brazell
14,371 PointsYep! You can even take out that print statement too
Ralph Nicholson
812 PointsRalph Nicholson
812 PointsThanks Jason. Definitely over thinking it!