Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial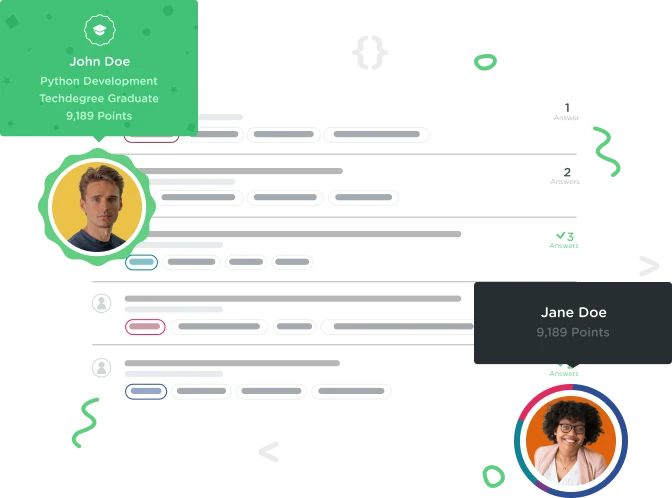

Fareez Ahmed
12,728 Pointssyntax for basic for loop in python
Hi,
Trying to work through some practice problems. I've studied JS and can do for loops there, but was wondering the best way to write for loops in Python. Here's an example problem:
var countdown = function(arr){
for(var i =arr.length-1; i>=0; i--){
console.log(arr[i])
}
}
What’s the best way to write the above code in python? I’m trying to understand how to do basic ‘for’ loops in python, counting arrays indices forwards, and backwards also.
def countdown(arr):
for idx in arr:
.....?
Could you also provide the 'translation' of something like this?
var array_pair_sum = function(arr, int){
for(var i = 0; i<arr.length - 1; i++){
for(var j = i + 1 ; j<arr.length; j++){
if((arr[i] + arr[j]) == int){return true;}
}
}
return false;
}
//example: array_pair_sum([2,3,5,7], 5) = true
3 Answers
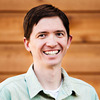
Joshua Ferdaszewski
12,716 PointsPython makes it really easy to work with each element in a list. Here is how you could print every item in a list.
# Print out every item in a list
items = [1, 2, 3, 4]
for item in items:
print(item)
That is it! Just a note, item
is just an arbitrary variable name, it can be any valid variable name you want. There are a few ways to print a list in reverse, here is how I would do it.
# Print a list in reverse order
items = [1, 2, 3, 4]
for item in reversed(items):
print(item)
Another way to reverse a list is to use the slice syntax: items[::-1]
This creates is a copy of the list starting at index 0 and ending at the last item, then steps through the list in reverse. You were getting an index error in your code because len()
returns the number of items in a list, not the index of the last item (which is what you want).
I hope this helps, again, I would recommend reading the documentation as there is a ton that lists can do in python.
Good Luck!

Fareez Ahmed
12,728 PointsThanks very much Joshua Ferdaszewski! Could you show me how to countdown/reverse without using the built-in reverse(maybe something similar to what I was trying to do with range/len above) and without copying the array using slice?
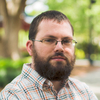
Kenneth Love
Treehouse Guest Teacheritems = [1, 2, 3, 4]
for item in items[::-1]:
print(item)
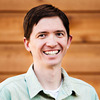
Joshua Ferdaszewski
12,716 PointsIt may be helpful for you to throw out what you know about JS looping when working with Python. The for loop in python is super easy and powerfull.
for x in some_iterable:
print x
This will assign each value in some_iterable
to the variable x, which you have access to inside the for loop. If you want to loop a specific number of times, just use range()
to create a list to iterate over.
for x in range(100):
print x
I would recommend checking out the documentation online, they probably explain it much better than I am :)
Good luck learning Python!

Fareez Ahmed
12,728 PointsI just edited the code which gives you an idea of what I know...so to print all elements/indices in an array, you'd do something like this?
# didn't use enumerate on purpose, trying to avoid built-in functions on purpose :)
list = [1, 2, 3, 4]
def countdown(lists):
for x in range(len(lists)):
print(lists[x])
countdown(list)
if I wanted to count/print backwards:
# I'm getting an error on this code: IndexError: list index out of range
list = [1, 2, 3, 4]
def countdown(lists):
for x in range(len(lists), 0, -1):
print(lists[x])
countdown(list)
But, this code, worked, why?
list = [1, 2, 3, 4]
def countdown(lists):
for x in range(len(lists)-1, -1, -1):
print(lists[x])
countdown(list)

Fareez Ahmed
12,728 PointsThanks Kenneth Love! So I'm deducing from your answer that using range/len to reverse an array is not really done or optimal in Python. Thanks!
Joshua Ferdaszewski
12,716 PointsJoshua Ferdaszewski
12,716 PointsInline code is very hard to read. Use the Markdown Cheatsheet, or follow the directions in this post