Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial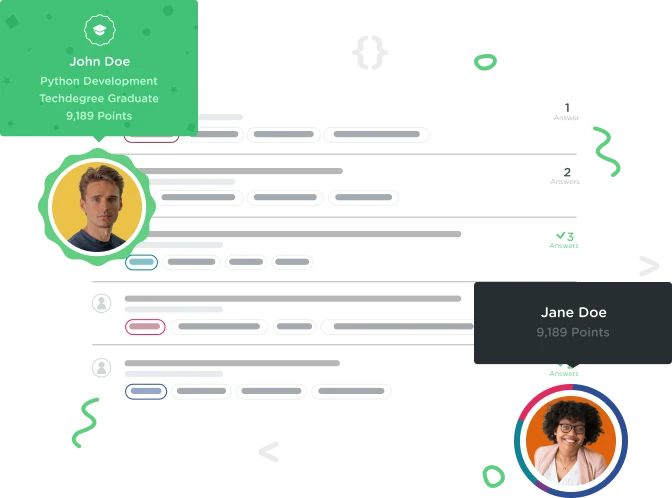

russellsoper
4,124 PointsTelling me I didn't present Treestory even though I am?
My main class
package com.teamtreehouse;
import java.util.Arrays;
import java.util.List;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String tmpl = prompter.promptForTemplate();
prompter.run(new Template(tmpl));
}
}
My Prompter class
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
this.mReader = new BufferedReader(new InputStreamReader(System.in));
this.loadCensoredWords();
}
private void loadCensoredWords() {
this.mCensoredWords = new HashSet();
Path file = Paths.get("resources", "censored_words.txt");
List words = null;
try {
words = Files.readAllLines(file);
} catch (IOException var4) {
System.out.println("Couldn't load censored words");
var4.printStackTrace();
}
this.mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List results = null;
try {
results = this.promptForWords(tmpl);
} catch (IOException var4) {
System.out.println("There was a problem prompting for words");
var4.printStackTrace();
System.exit(0);
}
String result = tmpl.render(results);
System.out.printf("Your TreeStory:%n%s", result);
}
public String promptForTemplate() {
try {
System.out.println("Please write the template");
String word = this.mReader.readLine();
System.out.println(word);
return word;
} catch (IOException var2) {
return "Something went wrong ";
}
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList();
Iterator var3 = tmpl.getPlaceHolders().iterator();
while(var3.hasNext()) {
String phrase = (String)var3.next();
String word = this.promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
System.out.printf("Enter your selection for %s%n", phrase);
String word;
for(word = this.mReader.readLine().toLowerCase(); this.mCensoredWords.contains(word); word = this.mReader.readLine().toLowerCase()) {
System.out.printf("%s is censored please pick another.%n", phrase);
}
return word;
}
}
My output
Please write the template
Testing __first prompt__ and then __second prompt__
second prompt is censored please pick another.
second prompt is censored please pick another.
Your TreeStory:
Testing pass_1 and then pass_2
Surely the TreeStory is presented at the end? Or am I misunderstanding what is wanted?

russellsoper
4,124 PointsEdited to add code.
1 Answer
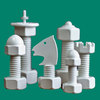
Steven Parker
231,275 PointsYou're really close! But when you check for a censored word, be careful to only change the case of the word for the purpose of comparison. Any non-censored word should be returned unmodified.

russellsoper
4,124 PointsGot it, always the little things. Thanks Steven!
Steven Parker
231,275 PointsSteven Parker
231,275 PointsYou need to provide more information (including your code) to replicate the issue.
Take a look at this video about Posting a Question.