Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial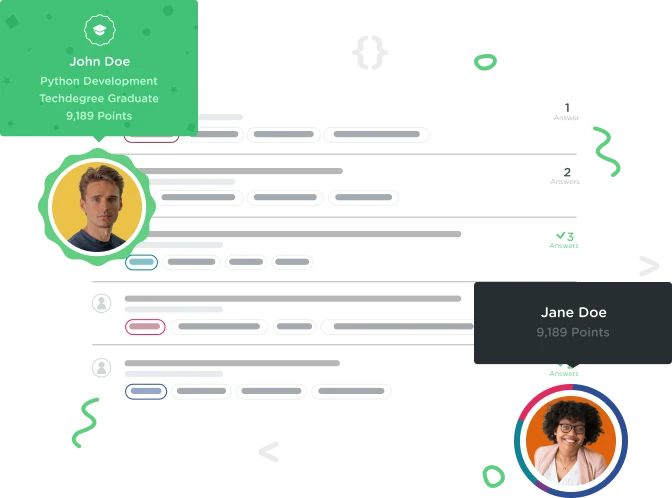

Alhagie Jobe
15,388 PointsThe for loop cycles over list items and applies a color to each item using the values stored in the colors array. For ex
The for loop cycles over list items and applies a color to each item using the values stored in the colors array. For example, the first color in the array ( #C2272D) is applied to the first list item, the second color (#F8931F) to the second list item, and so on. Complete the code by setting the variable listItems to refer to a collection. The collection should contain all list items in the <ul> element with the ID of rainbow.
var listItems = document.querySelectorAll("#rainbow");
var colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
for(var i = 0; i < colors.length; i ++) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html>
<head>
<title>Rainbow!</title>
</head>
<body>
<ul id="rainbow">
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="js/app.js"></script>
</body>
</html>
2 Answers
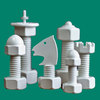
Steven Parker
229,732 PointsThe instructions say "The collection should contain all list items in the <ul> element with the ID of rainbow."
The selector used in the code here is setting the variable to the "rainbow" ul
itself instead of the li
items within it.
Hint: this might be a good place for a descendant selector or a direct child selector.

Daven Hietala
8,040 PointsI was able to find the answer on this thread..
"dallas kelley on May 24, 2018 While document.getElementsByTagName('li'); works for this particular problem it wouldn't work if you had more than one list that had different IDs. For example document.getElementsByTagName('li'); would select all <li> tags in a list that may not need to be selected where as document.querySelectorAll("ul#rainbow > li"); will only select <li> tags inside the UL with the ID: rainbow."
Good luck!
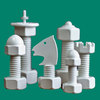
Steven Parker
229,732 Points The solution suggested in that other question will work, but it has excessive specificity. When referring to an element by ID, you don't also need the tag name.
Marcelo Pereira
12,169 PointsMarcelo Pereira
12,169 Pointsi already do that and still tell: There was an error with your code: TypeError: 'undefined' is not an object (evaluating 'listItems[i].style')
Steven Parker
229,732 PointsSteven Parker
229,732 PointsYour issue is probably earlier in the code. Try creating a new question where you can put your complete code and perhaps someone can help spot it.