Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial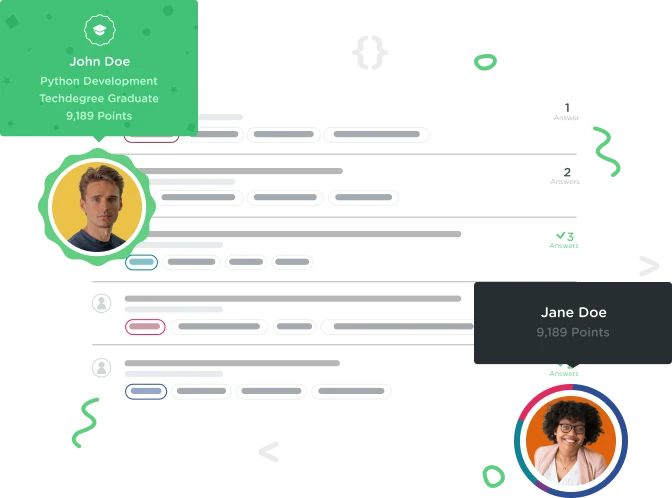

Morgan Strnad
14,292 PointsWhat am I suppose to do??
The objective is: Imagine you are writing a task management application that allows a user to track a list of tasks to complete. While testing your application, you receive an error saying that Thymeleaf cannot access the complete property in the expression ${task.complete}. This is due to an error in the Task model class given below. Correct the model class to fix the error. (Hint: Thymeleaf doesn't access the complete field value directly)
I am lost, please help!
package com.teamtreehouse.todo.model;
import java.time.LocalDateTime;
public class Task {
private String description;
private LocalDateTime dueDateTime;
private boolean complete;
public Task(String description, LocalDateTime dueDateTime) {
this.description = description;
this.dueDateTime = dueDateTime;
this.complete = false;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public LocalDateTime getDueDateTime() {
return dueDateTime;
}
public void setDueDateTime(LocalDateTime dueDateTime) {
this.dueDateTime = dueDateTime;
}
public void setComplete(boolean complete) {
this.complete = complete;
}
}
2 Answers
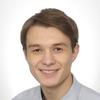
Sergey Podgornyy
20,660 PointsYou need to add getter
function to get private
property. Just add the following method to your class:
public boolean getComplete() {
return complete;
}

Morgan Strnad
14,292 PointsChris Ramacciotti Can you help me with this?
Morgan Strnad
14,292 PointsMorgan Strnad
14,292 PointsThanks, Sergey Podgornyy, now can you help me with this challenge please?
https://teamtreehouse.com/community/bummer-no-add-method-detected-that-accepts-one-parameter-of-type-contact-and-that-returns-a-string
Sergey Podgornyy
20,660 PointsSergey Podgornyy
20,660 PointsYou are welcome
Also, I have answered on your question on second link