Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial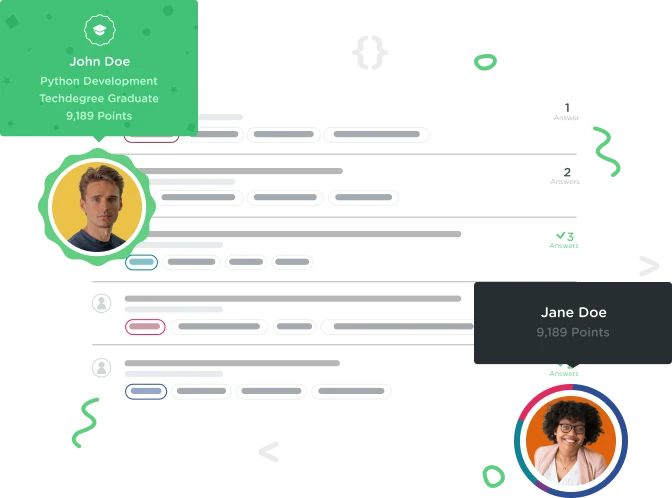

vikas pal
11,563 Pointswhat is self?
Please tell me what is self in python. Why we use it as a argument in function.what change it make in the code.Please explain with an example. Thank you Vikas

vikas pal
11,563 Points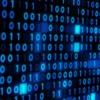
Alexander Davison
65,469 PointsSorry...
It is extremely hard to explain, and it is really confusing when you don't get it (I still don't fully understand, but I sort of understand).
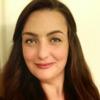
Jennifer Nordell
Treehouse TeacherHi there! Please refer to the amazing explanation by Chris Freeman
3 Answers
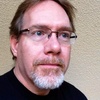
Chris Freeman
Treehouse Moderator 68,426 PointsWhen the class is instantiated to create a unique instance, it is given a unique ID (its address in memory). This ID is used to refer to this instance. The purpose of a class method is to manipulate the instance attributes or produce a result based on the instance attributes. The question is how to access the instance attributes from within the method and differentiate them from local variables within the method.
For attributes that belong to the class instance, they are referenced in compiled code by the instance ID, a dot, and the attribute name. But since we don't know the ID ahead of time, a placeholder variable is used. While this placeholder could be called almost anything, the convention in Python is to use the variable self.
Using this code as a reference, the id()
function is used to get the unique ID of an object:
class NewClass():
attr1 = 0
attr2 = "another attribute"
#
def method1(self):
print("inst ID: ", id(self))
print("inst attr1 ID: ", id(self.attr1), "inst attr1: ", self.attr1)
print("inst attr2 ID: ", id(self.attr2), "inst attr2: ", self.attr2)
attr1 = "local"
print("local attr1 ID: ", id(attr1), "attr1 value: ", attr1)
class AltClass():
attr1 = 1
attr2 = "nothing"
Executing method1()
shows the instance ID and the attribute IDs which are different from the local variable ID.
>>> nc = NewClass()
>>> nc.method1()
inst ID: 741831317432
inst attr1 ID: 1604767440 inst attr1: 0
inst attr2 ID: 741831297904 inst attr2: another attribute
local attr1 ID: 741831317240 attr1 value: local
Compare the instance ID derived from self
, above, to the ID of the instance directly:
>>> id(nc)
741831317432
They are the same!
As mentioned in the StackOverflow references below, using the instance method
nc.method1()
is syntactic sugar of the long form where the instance is passed explicitly to the class method.
NewClass.method1(nc)
These produce the same result
>>> nc.method1()
inst ID: 741831317432
inst attr1 ID: 1604767440 inst attr1: 0
inst attr2 ID: 741831297904 inst attr2: another attribute
local attr1 ID: 741831317240 attr1 value: local
>>> NewClass.method1(nc)
Inst ID: 741831317432
inst attr1 ID: 1604767440 inst attr1: 0
inst attr2 ID: 741831297904 inst attr2: another attribute
local attr1 ID: 741831317240 attr1 value: local
So... In the end, "self" is simply a placeholder for the ID of the current instance of the class.
You might ask: How does the instance method keep track of the value of self
when writing nc.method1()
? It is stored in a local variable nc.method1.__self__
:
>>> id(nc.method1.__self__)
741831317432
Now to bend your brain some, you might ask, can any instance be passed in when using the long form? Yes, it's possible, but a bad practice. If you look at the AltClass
, it has the same attributes attr1
and attr2
. What would happen if I passed an instance of AltClass
to NewClass.method1()
?
>>> ac = AltClass()
>>> ac.attr1
1
>>> ac.attr2
'nothing'
>>> id(ac)
741831316816
>>> id(ac.attr1)
1604767472
>>> id(ac.attr2)
741831316848
>>> NewClass.method1(ac)
inst ID: 741831316816
inst attr1 ID: 1604767472 inst attr1: 1
inst attr2 ID: 741831316848 inst attr2: nothing
local attr1 ID: 741831317240 attr1 value: local
Since self
gets assigned to the ac
instance which has the same expected attributes the method runs ok.
Note: Never code like this! It is only a twisted example of how the "self" can point to any instance.
Referenes: StackOverflow1, StackOverflow2
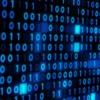
Alexander Davison
65,469 PointsThis is just a simple answer... (this wasn't written by me, but was written by Nathan Barnes 5 months ago).
The 'self' argument means the instance acting on itself, so when you create an object and call a method that uses the self argument within its body, then the actions within that method will be applied to the object calling the method.
And my explanation (if that is just gibberish to you):
The self
argument refers to the instance. If you are writing a method and you wanted to call one of the functions that are in the same class, you can just do self.method()
instead of Class.method
. (Little notes: You only can do this if the method/attribute is part of the same class.)
Good luck! ~alex

vikas pal
11,563 PointsThank you to all now i know what is self and why we use it.Really great help.Chris and Alexander thank you very much.
vikas pal
11,563 Pointsvikas pal
11,563 PointsKen Alger