Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial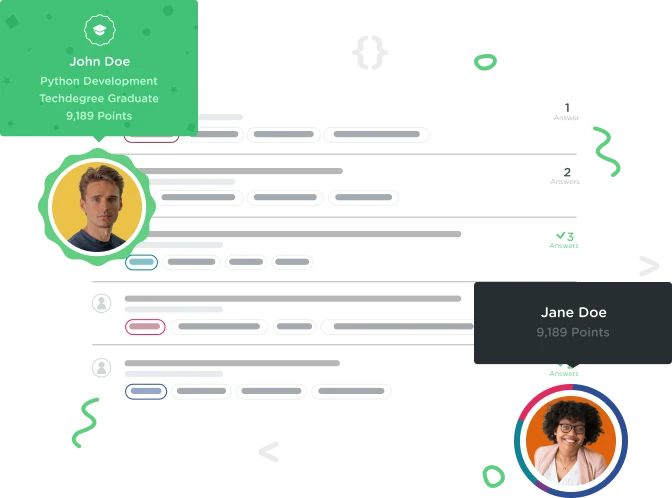

Matjaž Vrečer
3,911 PointsWhat is wrong with this?
def discount(price, amount): return price - price * (amount/100)
from functools import partial discount_10 = partial( discount, amount=10 ) discount_25 = partial( discount, amount=25 ) discount_50 = partial( discount, amount=50 )
prices_10 = map( discount_10, prices ) prices_25 = map( discount_25, prices ) prices_50 = map( discount_50, prices )
prices = [
10.50,
9.99,
0.25,
1.50,
8.79,
101.25,
8.00
]
def discount(price, amount):
return price - price * (amount/100)
from functools import partial
discount_10 = partial( discount, amount=10 )
discount_25 = partial( discount, amount=25 )
discount_50 = partial( discount, amount=50 )
prices_10 = map( discount_10, prices )
prices_25 = map( discount_25, prices )
prices_50 = map( discount_50, prices )
2 Answers
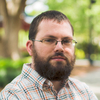
Kenneth Love
Treehouse Guest TeacherYep, just had a slightly too agressive regex on there. Your original post now passes.
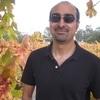
Kourosh Raeen
23,733 PointsI took away the spaces around the parameters in your code and it passed:
prices = [
10.50,
9.99,
0.25,
1.50,
8.79,
101.25,
8.00
]
def discount(price, amount):
return price - price * (amount/100)
from functools import partial
discount_10 = partial( discount, amount=10 )
discount_25 = partial( discount, amount=25 )
discount_50 = partial( discount, amount=50 )
prices_10 = map(discount_10, prices)
prices_25 = map(discount_25, prices)
prices_50 = map(discount_50, prices)

Matjaž Vrečer
3,911 PointsThank you!
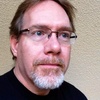
Chris Freeman
Treehouse Moderator 68,457 PointsWhile I agree with this correct solution, I see this as a challenge checker error since it is only a style error not a syntax error.
Tagging Kenneth Love to review the checker to allow the spaces or provide a PEP-8 related error message.
Chris Freeman
Treehouse Moderator 68,457 PointsChris Freeman
Treehouse Moderator 68,457 PointsThanks for the continuous quality improvement!