Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial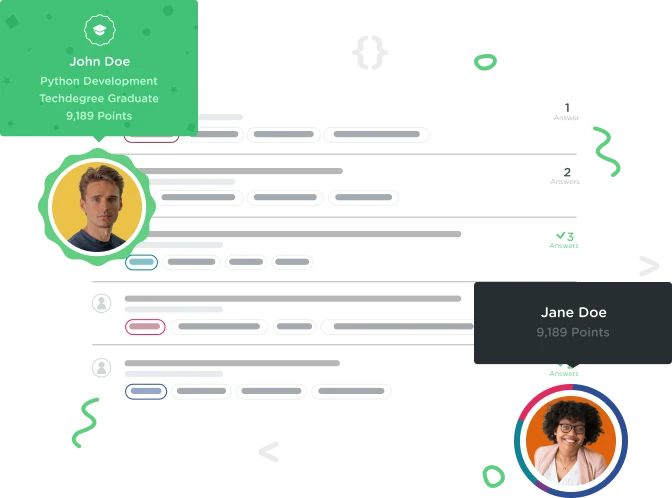
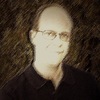
Jason Anders
Treehouse Moderator 145,860 PointsWhat little thing am I missing? "Bummer! Hmm, didn't get the right success message from `ArticleCreate'
Challenge Link (cuz "Get Help" button still doesn't work) -> https://teamtreehouse.com/library/django-classbased-views/customizing-classbased-views/custom-mixin
Task 4 of 4
articles/views.py
from django.contrib.auth.mixins import LoginRequiredMixin
from django.core.urlresolvers import reverse_lazy
from django.views import generic
from . import mixins
from . import models
class ArticleList(generic.ListView):
model = models.Article
class ArticleDetail(generic.DeleteView, generic.DetailView):
model = models.Article
template_name = 'articles/article_detail.html'
class ArticleCreate(LoginRequiredMixin, mixins.SuccessMessageMixin, generic.CreateView):
fields = ('title', 'body', 'author', 'published')
model = models.Article
success_message = "Article created!"
class ArticleUpdate(LoginRequiredMixin, mixins.SuccessMessageMixin, generic.UpdateView):
fields = ('title', 'body', 'author', 'published')
model = models.Article
def get_success_message(self):
obj = self.get_object()
return "{} updated!".format(obj.title)
class ArticleDelete(LoginRequiredMixin, generic.DeleteView):
model = models.Article
success_url = reverse_lazy('articles:list')
class ArticleSearch(generic.ListView):
model = models.Article
def get_queryset(self):
qs = super().get_queryset()
term = self.kwargs.get('term')
if term:
return qs.filter(body__icontains=term)
return qs.none()
articles/mixins.py
from django.contrib import messages
class SuccessMessageMixin:
success_message = ""
def get_success_message(self):
return self.success_message
def form_valid(self, form):
message.success(self.request, get_success_message)
return super().form_valid(form)
I feel everything is correct... but still there must be one little mistake I'm blind to. :)
4 Answers
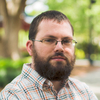
Kenneth Love
Treehouse Guest TeacherYour mixin never calls its own get_success_message
method, it just references it. You need to actually call it (with parentheses). Next to last line in your pasted code.
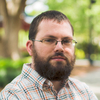
Kenneth Love
Treehouse Guest TeacherYou'll also need self
in there...
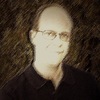
Jason Anders
Treehouse Moderator 145,860 PointsBut the error I'm getting is for the first 'hard-coded' string not being correct. Is there a method call there?
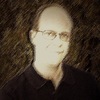
Jason Anders
Treehouse Moderator 145,860 PointsGot it!!! Thanks Goodness. Thank-you Kenneth Love!
It was that, but I was also missing an 's' on "messages" in messages.success...
I can finally go to sleep!

Nicolas Baranowski
1,992 PointsHi Kenneth Love ,
I'm actually in the same situation as Jason was: I'm not calling get_success_message
method.
Could you please be a bit more specific on how I should do it?
Thanks :)
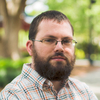
Kenneth Love
Treehouse Guest TeacherNicolas Baranowski the form_valid
method needs to call the get_success_message
method to get the success message.
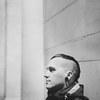
Martynas Zilinskas
22,087 PointsSorry what confusing in this challenge that it passes in Challenge 3 without this(so you assume that everything is fine here): def form_valid(self, form): message.success(self.request, self.get_success_message()) return super().form_valid(form)
And in Challenge 4 it looks like you've been asked just to edit articles/views.py
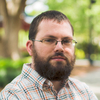
Kenneth Love
Treehouse Guest TeacherThat snippet you included looks fine except it's messages
. I'll double check the validator for that.
I added a few more checks to the validator for that step. Hope that helps!
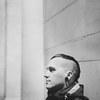
Martynas Zilinskas
22,087 PointsThanks, Kenneth!!!
Love your challenges ;)
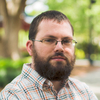
Kenneth Love
Treehouse Guest TeacherMistake in the code, you never called your method.
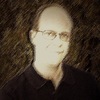
Jason Anders
Treehouse Moderator 145,860 PointsKenneth Love Could you elaborate juuuust a bit more... :)
I think I've been staring at this for too long today.
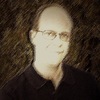
Jason Anders
Treehouse Moderator 145,860 PointsI'm not sure which method you're referring to? :(
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsSo, Kenneth Love ... a mistake in the challenge or a mistake in the code?