Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial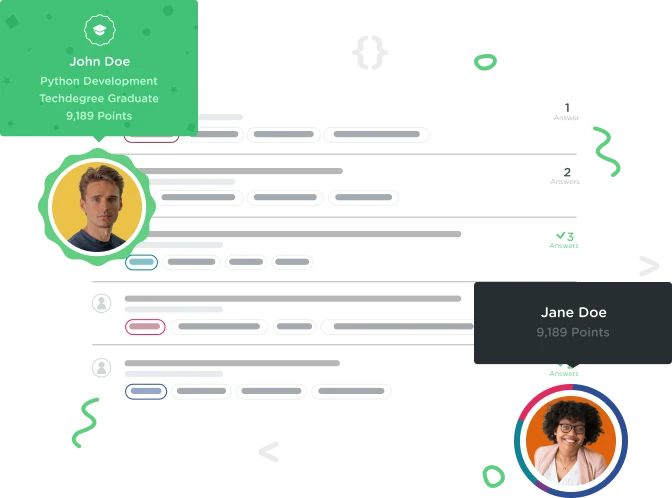

Phillip Kazanjian
6,273 PointsWhy can't I use an arrow function?
Hi:
I do not understand why I receive an exception when using an arrow function. Are arrow functions and traditional function declarations not the same?
This works as expected with the traditional function declaration
const myString = { string: "Programming with Treehouse is fun!", countWords: function () { return this.string.split(' ').length; } }
I receive 'string is undefined' when using the arrow function
const myString = { string: "Programming with Treehouse is fun!", countWords: () => { return this.string.split(' ').length; } }
2 Answers
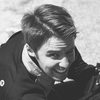
Brandon Leichty
Full Stack JavaScript Techdegree Graduate 35,193 PointsHello Phillip,
You can find my answer to a similar question here.
I'll re-share some of what I wrote below.
The short answer is that since you're using an arrow function, "this" is bound to the Window object instead of the myString.
Try running this code and see what you get:
const myString = {
string: "Programming with Treehouse is fun!",
countWords: () => {
return this
}
};
You'll notice that what "this" is referring to inside your arrow function is the Window object. So what you're really returning is Window.string.split(" ").length;. Which doesn't exist.
You could rewrite your arrow function like this:
const myString = {
string: "Programming with Treehouse is fun!",
countWords: () => {
return string.split(" ").length;
}
};
Here are some additional resources that might help:
This is the first article I'd suggestion reading. Specifically the Main benefit: No binding of βthisβ section towards the bottom of the article. Here's an excerpt:
In classic function expressions, the this keyword is bound to different values based on the context in which it is called. With arrow functions however, this is lexically bound. It means that it uses this from the code that contains the arrow > function.
"this" is a very confusing and misunderstood aspect of JavaScript. If you want to go deeper into how it works, Kyle Simpson has written a book (available for free on GitHub) that goes deep into how "this" works.
There are four rules to determine the context of "this":
Is the function called with new (new binding)? If so, this is the newly constructed object. Example: var bar = new foo()
Is the function called with call or apply (explicit binding), even hidden inside a bind hard binding? If so, this is the explicitly specified object. Example: var bar = foo.call( obj2 )
Is the function called with a context (implicit binding), otherwise known as an owning or containing object? If so, this is that context object . Example: var bar = obj1.foo()
Otherwise, default the this (default binding). If in strict mode, pick undefined, otherwise pick the global object. Example: var bar = foo()
Number four above is where your "this" falls under. Which is why it returns the Window/global object.
Treehouse also has a workshop on arrow functions that I'd recommend checking out if you haven't already done so.
I hope this helps! If you have any additional questions, please let me know!
Happy coding! Brandon

Phillip Kazanjian
6,273 PointsWow! Thank you for taking the time to provide that answer. I sincerely appreciate it! Your answer makes sense to me. I'll be sure to check out TreeHouse's arrow function module. I'll save that reading on GitHub for later. :)