Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial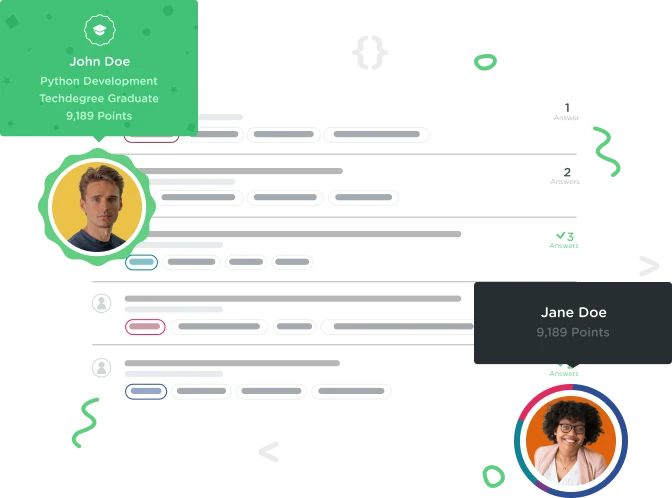

Ben Os
20,008 PointsWhy do we even need Callbacks?
If callbacks are actually """a function statement written inside the argument parenthesis of another function, that then can be called later in the program"""
Than, it is unclear to me why we need to use them and just write functions one after the other...
Why putting it as an argument to function A instead of putting it under it as function B and then call it when we want?

Ben Os
20,008 PointsHi Joel... Sadly I don't have a code to show as I tried to read on the concept and didn't quite get its purpose. I did hear Guil Hernandez just reminding the concept and saw it in a video by Aisha blake using it on jQuery but it wasn't clear to me why do we need callbacks and the answers below talking about async code (which I don't remeber Guil and Aisha talked about) made me even more confused. I talked with a programmer on this on phone and he told me that in the most general way, a callback is when we state a function inside parenthesis of another function and then call it later somewhere in the code (maybe he meant we call the function that hosts it and it will run as well, maybe async, given the answers I was given below). I feel I miss the concept in general and wrote the quote trying to reflect my understanding of this concept but I feel I still miss the general concept. I think a video on the concept itself (that also touches sync vs async) could be so helping to so many...

Ben Os
20,008 PointsAnyway, Joel, maybe another explanation will help me understand it even better as it would address my current understanding that someone else might have...
4 Answers

Nathan Dalbec
17,111 PointsWell, callbacks are necessary for asynchronous programming, including waiting for a user request, making a request to another server and doing something with the response, loading a file, etc. If you used a function synchronously instead of in a callback, then the function would be called before you get the data that the function needs and therefore it wouldn't work. As to the second part of your question, you could declare a function and pass that as the callback instead of passing in an anonymous function, both will work just fine.
function asyncFunction (callback) {
setTimeout(callback, 1000, 'foo'); // passes foo to the callback
}
//This works
asyncFunction(function(bar) {
console.log(bar)
});
// this also works
function bat(bar) {
console.log(bar)
}
asyncFunction(bat)
On the other hand, this does not work:
var foo;
function asyncFunction () {
setTimeout(function() {
foo = 'bar';
}, 1000);
}
function bat() {
console.log(foo);
}
asyncFunction();
bat(); // logs out undefined

andren
28,558 PointsWe need them because a lot of operations in JavaScript are asynchronous, or put a bit more simply they don't actually stop the program from continuing until they are done like you are probably used to. But instead just run in the background while the rest of the code continues executing.
If you wanted to fetch some data from a server for instance (which could takes an unknown amount of time) it would be incredibly inefficient for your program to just freeze completely while it waited for that data to be fetched. So instead of doing that it's common to just run the fetching task in the background.
This means that if you have two functions in a row with function A being asynchronous then function B will be executed while function A is still running. In that case if function B depends on data that function A is fetching you will run into problems.
This problem is solved with callbacks. With a callback you can guarantee that function B is only called after function A is finished with its thing because function A is actually the one responsible for calling function B.
Asynchronous functions also aren't the only use of callbacks, but they are the most common and useful use of them that I can think of at the top of my head.

Richmond Mensah
2,445 Pointsgood explanation

Joel Kraft
Treehouse Guest TeacherBen,
The fundamental reason for a callback is to run code in response to an event. These events could be user-generated, like mouse clicks or typing for example. With a callback you can tell your program, "every time the user presses a key on the keyboard, run this code". To register a callback function for an event, you need to be able to pass it to another function, which is responsible for binding the event and callback together (i.e. make it so the callback executes, or runs, when the event occurs).
For instance, let's say you want to execute the function "callback
" when the user clicks on the body of a webpage. In vanilla JS, you could write:
const body = document.getElementsByTagName('body')[0];
function callback() {
console.log('Hello');
}
body.addEventListener('click', callback);
In this case, we are passing a function, "callback
" to another function "addEventListener
". When "addEventListener
" runs, it registers "callback
" with the click event.
Notice there are two different ways JavaScript is being run here. The script runs once when the page loads. Here is what happens during that execution:
- The "
body
" constant is declared, and a value is assigned to it. - The function "
callback
" is declared, but not executed. - The method (i.e. function) "
addEventListener
" is executed. (callback
is passed in toaddEventListener
. Again,callback
is not executed yet, onlyaddEventListener
is. Its job is to tell the browser to runcallback
when the click event occurs on the body.)
Then, the other time JavaScript is run is when an event occurs, namely the click event. This is when the function callback
is finally executed. It has only one line, console.log('Hello');
. So that line executes when the body is clicked, and "Hello" is logged to the console. This can happen more than once, namely whenever the body is clicked.
Using jQuery might look like this:
function callback() {
console.log('Hello');
}
$('body').on('click', callback);
Notice it's very similar, but we're passing "callback
" to a function called "on
", instead of "addEventListener
". In either case, you're handing your callback function over to another function, which is taking care of setting up the event listener for you.
There are other events besides user events. They could be called system events. The events that Nathan Dalbec and andren mention in their answers would fall into this category. In one, a callback is executed in response to a certain amount of time passing. In another, a callback is executed in response to a server returning data. The same basic thing is happening. An event occurs, and a callback function runs in response.
Does this make sense?

Ben Os
20,008 PointsHi Joel, I read your answer carefully and slowly (I thank you for it dearly). It sure does makes sense yet sadly I think I still miss it. The distinction you make between user-triggered events or system-triggered events helps me to gain better understanding of program processes, especially the examples of executing the callback after some time passing or after the servers returns some data.
Yet I think my problem is with the phrase "A callback is executed"; I think I miss what exactly is executed...
Is saying "a callback is executed" EQUALS "executing a function that includes another function as as its second-or-later argument"?
I also asked myself if it always deals with event handling...
I am sorry if It seems I overthink this. I really feel I miss it.

Joel Kraft
Treehouse Guest TeacherI updated my answer, which hopefully makes it clearer. The callback function is the function being passed in to the other function (the one responsible for registering the callback to respond to events). When the callback executes, "Hello" is logged to the console. Let me know if you're still confused. :)

Ben Os
20,008 PointsThank you so so much Joel! I am convinced I now have a basic understanding of it:
In the begining I thought we declare or express the callback function as a parameter of the second function, but from your answer I now know that we declare or express it as a standalone function, and then just calling it back, as an argument in the second function (which is either the built-in method .addEventListener or .on in jQ).
I now understand that calling it back as a second argument after either .addEventListener or .on, allows, thanks to the logical nature of the Javascript language, to initiate our called-backed response, after a relevant event was triggered.
I must say that I, as a Treehouse student would be so happy to see a film that explains more on the logics of how actually the fact that this is a second argument makes it to stay there and "amubsh" events, by means of turing completness logic and Javascript engine inside a browser.
Thank you again for all the help Joel!

Joel Kraft
Treehouse Guest TeacherGlad to hear you're starting to understand it better. Like many things in JavaScript, working on projects will help you solidify and expand your knowledge and understanding. Keep up the great work!
Joel Kraft
Treehouse Guest TeacherJoel Kraft
Treehouse Guest TeacherHi Ben, where are you getting the quote? Is this question in response to a particular treehouse video? Callbacks can be used in a several different ways, so it might be helpful to know how you're seeing them used. If possible, post the code you're working on too. :)