Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial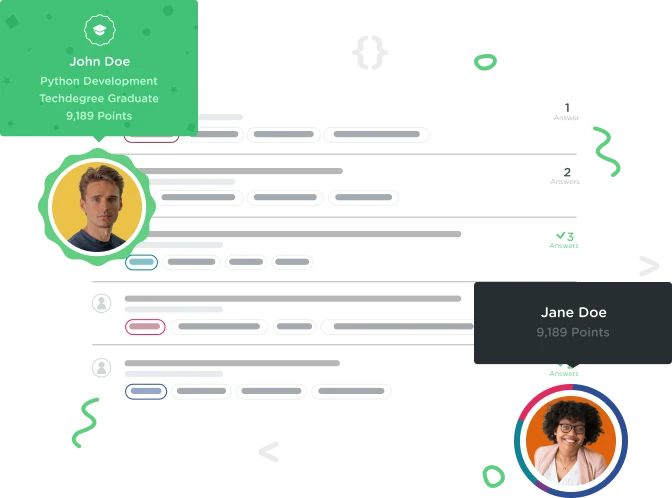

ALEKSANDAR TODOROVSKI
Courses Plus Student 8,508 PointsWhy double is used instead of int as a type for the arguments in the methods for the numbers 3 and 5 ?
using System;
class Program { static void Add(double first, double second) { Console.WriteLine(first); Console.WriteLine(second); Console.WriteLine(first + second); }
static void Subtract(double first, double second)
{
Console.WriteLine(first);
Console.WriteLine(second);
Console.WriteLine(first - second);
}
static void Main(string[] args)
{
Add(3, 5); // => 8
Add(10.5, 7.2); // => 17.7
Subtract(9, 3); // => 6
Subtract(21.3, 7.1); // => 14.2
}
}
3 Answers
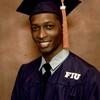
Dane Parchment
Treehouse Moderator 11,077 PointsThis is because it is a generic addition/subtraction function. We aren't trying to limit the number of...well...numbers that we could add/subtract together. If we were to limit the data types to integers we wouldn't be able to do things like:
Add(1.1, 2)
Subtract(3.6, 2.8)
Like you saw in the above answer, we can still provide integers, they just get an appended .0
added to it
So like my example
Add(1.1, 2)
Is read as
Add(1.1, 2.0)
Hope that helps you understand why

kevin hudson
Courses Plus Student 11,987 PointsHelps but please... please just stick to low-level teaching instead of straight out confusing a new developer.
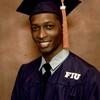
Dane Parchment
Treehouse Moderator 11,077 PointsThis is very low level, can you explain how this was confusing for you?

kevin hudson
Courses Plus Student 11,987 PointsDane Parchment I completely understand why double is used here, I meant to direct this to the course instruction. Newcomers that are on a learning path doesn't need the extra edge cases to worry about until an appropriate time when this is necessary. Teamtreehouse courses quite often add extra information to their instruction that can confuse the audience.
For this example, I would have gone with int for the easy explanation. The purpose, in which, is the desired result that I at least expect.
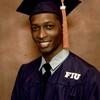
Dane Parchment
Treehouse Moderator 11,077 PointsAh okay, that makes sense. For some reason I thought you were directing the comment towards my answer.
ALEKSANDAR TODOROVSKI
Courses Plus Student 8,508 PointsALEKSANDAR TODOROVSKI
Courses Plus Student 8,508 PointsYes . Thank you sir !
Scott George
19,060 PointsScott George
19,060 PointsDane Parchment "This is because it is a generic addition/subtraction function" This is what I thought, after thinking about it for a moment. It seems very simple and clear. Thanks :)