Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial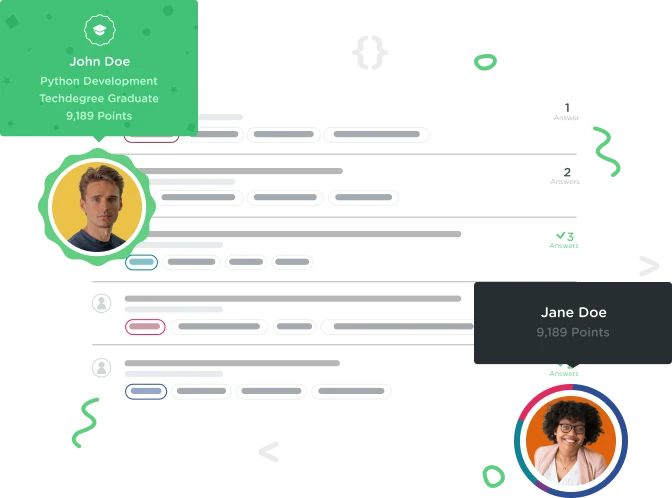
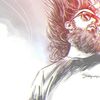
Aaron Eckhart
3,341 PointsWhy would a plist for constants be better for a game with multiple levels? Can anyone expound on that for me? Thanks
.
4 Answers
Mike Baxter
4,442 PointsA plist is really simple to understand once you know the following objects: NSArray, NSDictionary, NSString, NSNumber. You can make a plist by clicking File > New File > Resource > Property List.
To read a plist, you'll want to make sure you have set up an NSMutableArray to read it into (so you can clear the array every time you load a new level, and then load it with new data). It's then just a couple lines of code to read in the data.
NSMutableArray *levelVariables = [[NSMutableArray alloc] init];
NSString *pathToPlist = [[NSBundle mainBundle] pathForResource:@"levels" ofType:@"plist"];
levelVariables = [NSArray arrayWithContentsOfFile:pathtoPlist];
I think the hardest part in all of this will be deciding what to put inside of that plist. (That is to say, architecting the data your game needs.) There's really no one way to do this, and every game does things differently. You just want to find something that makes sense with what you're doing. And remember to avoid reading from the plist more than once per level. (i.e., don't call "arrayWithContentsOfFile" more than once per level, just reuse data inside the NSMutableArray.)
Mike Baxter
4,442 PointsA plist gives you a nice way to order information. You can very easily create NSArrays, which can contain numbers and strings, NSDictionaries, or even NSData. Numbers can be your constants for things like damage multipliers, health points, and so on. You'll probably spend a lot of time tweaking those numbers and strings, so it would be nice to have them all in one place. I think in the long run a plist would make things easier to compare, especially since you can see the branches of an array. Imagine you have an array, with one entry for each level. Inside of each row (level) you could put a secondary array of the variables you have for that level.
When you read your plist into the app, you are going to have to read it in as an array (or a dictionary if your top-level of the plist is a dictionary). You'll probably want to do this only once per level, and save that in a local NSMutableArray. (You REALLY don't want to be loading in the plist every time you have to make a draw call.) When the next level loads, clear the array. You'll probably want to make strong use of NSDictionary, such as @{ @"damageMultiplier": @500 };
Basically, the plist is useful because its a nice visual way to make things work. There might be some other advantages I'm not aware of.
I hope this helps!
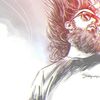
Aaron Eckhart
3,341 PointsHey thanks a lot Mike, definitely helped. I haven't worked with plist's beyond anything rudimentary. Is there a good tutorial on here or anywhere else that would give me a deeper understanding of how to manipulate and implement a plist? Thanks again!
Aaron
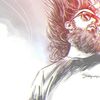
Aaron Eckhart
3,341 PointsYou're the man, thanks a lot.
Mike Baxter
4,442 PointsGlad I can help! If you want a much deeper look into making games, check out "Game Engine Architecture" by Jason Gregory. It's probably the best book on programming games. Only a chapter or two of it will be useful if you're making stuff with SceneKit, and it definitely won't talk about SceneKit, but there are good paradigms to follow. So probably check it out from a library rather than buy it. It's surprisingly difficult to find out how people architect their games, and I think the reason for that is because most every game does it differently. Gregory's book is good because it sort of lets you in on the secretβthe guy has first-hand knowledge building game engines and working with some of the top studios, so he offers lots of information on how things are done.