Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- Environments 4:50
- Accessing Environment Variables 3:50
- Environments and Their Variables 5 questions
- Setting up the Project and Package 5:06
- Managing Credentials with Environment Variables 2:39
- Using Environment Specific Settings 4:18
- PHP dotenv Package 5 questions
- Complex Data Types 6:21
- Additional Considerations 5:50
- Learning Environment 0:47
- More Data 5 questions
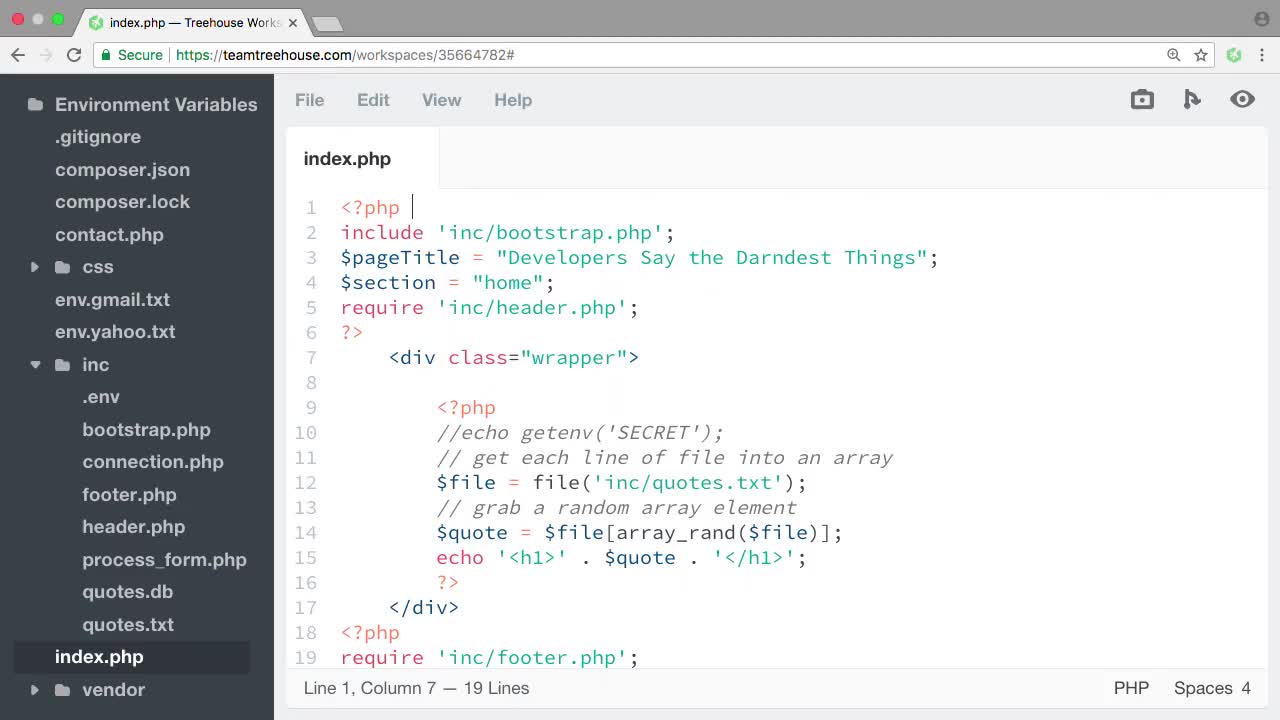
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
There are MANY different ways to handle switching out the data source. Since this course is on Environment Variables, I'm going to show you ONE way to use environment variables by storing a complex data type using JSON.
More Explanation
This video went quickly though a number of items you should already be familiar with. If you would like a refresher, please check the following resources.
- File Handling with PHP - where we cover Reading JSON and Writing JSON
- putenv() function - Sets the value of an environment variable. The environment variable will only exist for the duration of the current request. At the end of the request the environment is restored to its original state. This can be useful for testing.
- PHP Arrays and Control Structures where we cover switch statements
- Integrating PHP with Databases where we cover PDO
Code Example
// set environment variable
putenv('REPOSITORY={"type":"sqlite","source":"inc/quotes.db"}');
//read environment variable into an object
$repository = json_decode(getenv('REPOSITORY'));
switch ($repository->type) {
case 'text':
$file = file($repository->source);
// grab a random array element
$quote = $file[array_rand($file)];
break;
case 'sqlite':
try {
//if you are working in workspaces you will most likely need to start with 'sqlite:/www/'
$db = new PDO("sqlite:" . $repository->source);
$db->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
$result = $db->query('SELECT quote FROM Quotes ORDER BY RANDOM() LIMIT 1');
$quote = $result->fetchColumn();
break;
} catch (Exception $e) {
echo "Error!: " . $e->getMessage();
}
default:
$quote = 'It works on my machine.';
}
Optionally you can decode JSON data into an associative array if you prefer, by setting the second parameter of the json_decode function to true.
$repository = json_decode(getenv('REPOSITORY'), true);
switch ($repository['type']) {
...
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up