Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
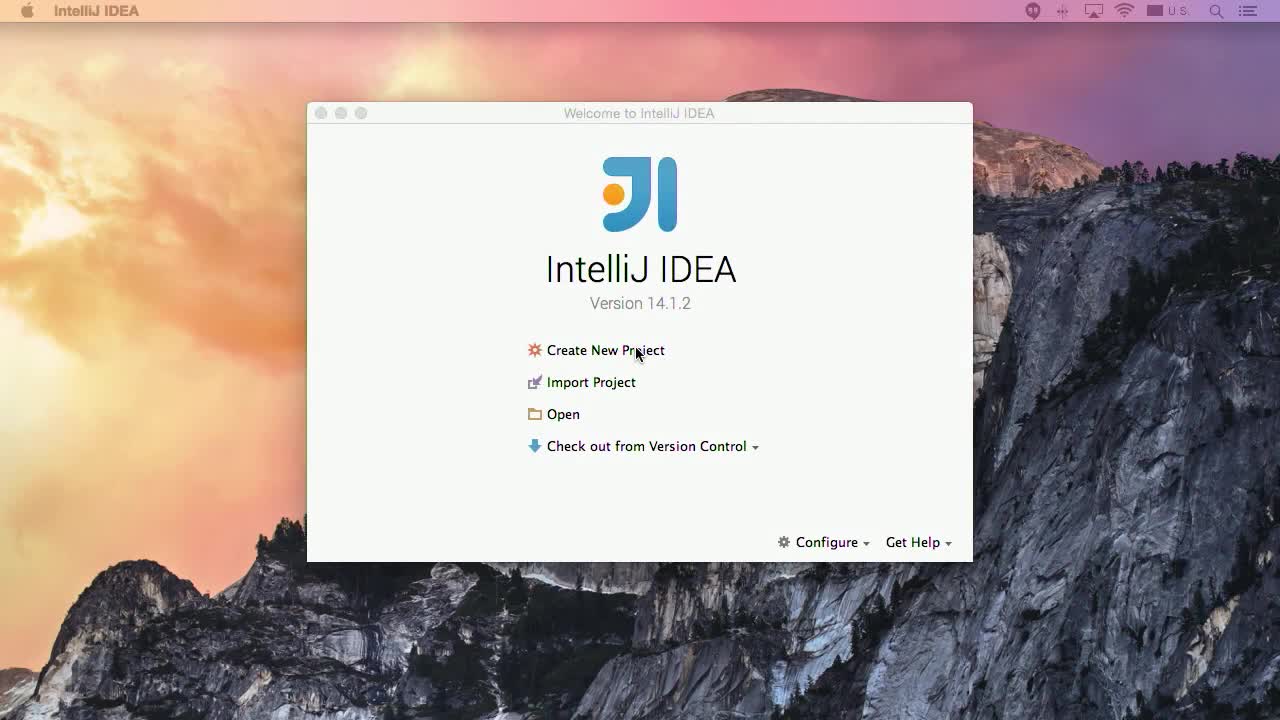
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Projects are the central concept of developing in IntelliJ IDEA. Let's explore how to create them and navigate around.
Note
The user interface for IntelliJ IDEA has changed since the filming of this video and the process for creating a new project is slightly different. Please refer to the Create Your First Project in IntelliJ IDEA instruction page for updated steps for creating a new project.
Code
package com.teamtreehouse;
import java.util.Set;
import java.util.TreeSet;
public class Systemizer {
public static void main(String[] args) {
System.out.printf("This is the classpath: %s %n",
System.getProperty("java.class.path"));
Set<String> propNames = new TreeSet<String>(System.getProperties().stringPropertyNames());
for (String propertyName : propNames) {
System.out.printf("%s is %s %n",
propertyName,
System.getProperty(propertyName));
}
}
}
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up-
Chance Edward
4,507 Points1 Answer
-
Cade Mell
1,544 Points0 Answers
-
Mustafa Khan
6,224 Points1 Answer
-
Geraldine Tanaka Munyoro
5,170 Points0 Answers
-
PLUS
Kenneth Phillips
Courses Plus Student 10,188 Points2 Answers
-
Michael Stedman
Full Stack JavaScript Techdegree Student 13,838 Points0 Answers
-
Christopher Mlalazi
Front End Web Development Techdegree Graduate 17,305 PointsEvent Log
2 Answers
-
Michel Bourgeois
731 Points2 Answers
-
Shafeeq Ahmed
6,058 Points1 Answer
-
Kshatriiya .
1,464 PointsSolved: Intellij auto completion/postfix completion not working
Posted by Kshatriiya .Kshatriiya .
1,464 Points1 Answer
-
Drew Warren
4,565 Points2 Answers
-
Greg Wienecke
20,765 Points2 Answers
-
Courtney Mahida
2,367 Points1 Answer
-
Kimberly Rice
11,656 Points3 Answers
-
Natasha Johnson
12,285 Points2 Answers
-
Gabriel E
8,916 Points3 Answers
View all discussions for this video
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up