Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Preview
Video Player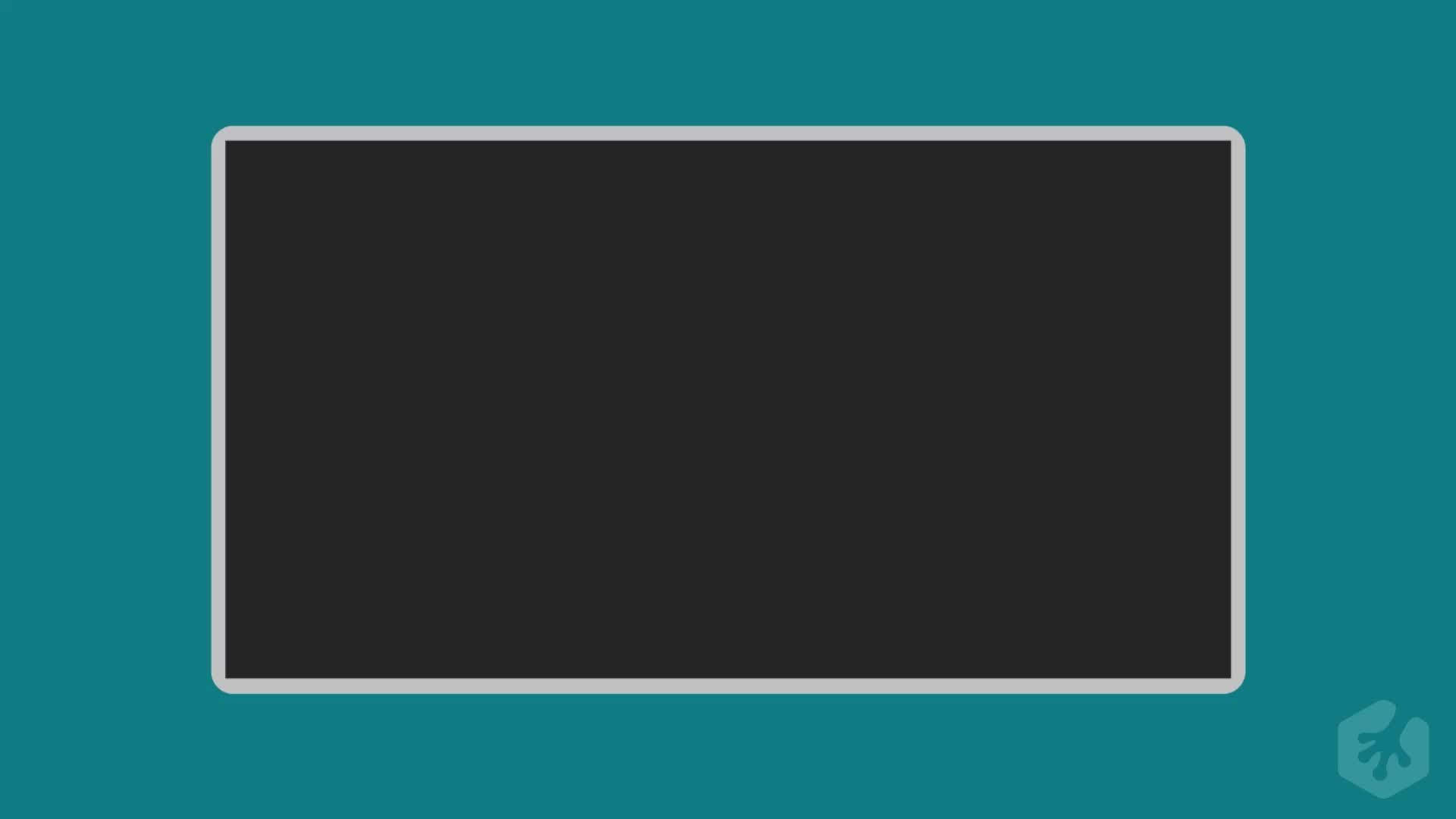
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Let’s take the loops from the last video and see how we can simplify them with list comprehension!
Resources
The Syntax
# list comprehension to produce a list
[expression for temp_var in iterable]
# list comprehension with .join() to produce a string
"".join([expression for temp_var in iterable])
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
In the last video, we looked at two
basic for loops and their syntax.
0:00
Now, I'll teach you how to use Python
list comprehension to make your code more
0:05
efficient.
0:10
We'll start with the list
comprehension syntax, as promised,
0:12
it's only one line, let's break it down.
0:16
These square brackets are used
to hold the values generated by
0:19
the list comprehension.
0:23
This should look quite familiar,
because Python lists are also held in
0:26
square brackets, for and in our
keywords that are part of the syntax.
0:31
These should also look pretty familiar
since they're part of the for
0:37
loop syntax as well.
0:42
Iterable, is the original list or
string that we want to iterate over.
0:43
Temp_var, is the temporary variable name
given to the value that we've iterated to.
0:49
Expression, is a valid block of code
that gets run and returns a value.
0:56
This is the action that is to be taken,
like are capitalizing of names.
1:03
Let's open up list_comprehension.py.
1:08
In there, you'll find our two
loops from the previous video,
1:12
as well as the list_comprehension
syntax template ready to go.
1:17
Let's turn our name capitalizing loop
into a one-line list comprehension.
1:24
First, let's uncomment the syntax.
1:30
We can either simply
delete this hash here, or
1:33
use a handy shortcut to toggle comments.
1:36
CMD + / on macOS or CTRL + / on Windows.
1:40
Then we'll start replacing parts of
the syntax template with the real deal.
1:46
We'll start with original iterable,
that's simply this list we've got here.
1:51
So I'll copy and paste and replace that.
1:56
Temporary variable, is simply this one,
2:00
that we've got in our loop,
nametag, so I'll copy and paste.
2:03
And finally expression, is simply this
one, is what changes to our nametag.
2:08
Now, you'll notice that this append syntax
isn't needed in the list comprehension
2:17
because the surrounding square
brackets are already in place
2:23
to append each return value from
the expression to the new_nametags list.
2:27
And here is our one line
compared to our four line loop.
2:34
Let's comment out our
original for loop and
2:38
then uncomment our print statement at
the bottom, make sure to save the file.
2:44
You can save the file by
going to File > Save or
2:50
simply using the handy shortcut CMD + S
for macOS or CTRL + S for Windows.
2:54
If your file is unsaved in workspaces,
you'll see this orange dot.
3:01
Once it is saved, the dot goes away.
3:06
Let's run list_comprehension.py now.
3:09
With Python3, 2, you'll see a list with
our names all capitalized as expected.
3:12
Just like we saw with the original for
loop, congrats,
3:21
you've just written your
first list comprehension.
3:24
Now let's try the same with our string,
the welcome banner.
3:29
We're gonna scroll up here to the loop,
uncomment the template and get replacing.
3:33
Original_iterable is our welcome string,
3:40
temporary_variable is the letter
that we used in the loop,
3:45
and expression is letter.upper,
3:50
where we uppercase every letter, great.
3:54
Let's comment out the loop and
uncomment the print statement.
3:58
Let's make sure to save and
then run the file.
4:04
Now another handy command line tip for
you.
4:07
You can press the Up arrow to go
back through your command history.
4:10
So I just pressed up here to get to
the command to run the file again.
4:14
But wait, that's not a string,
that's a list of individual characters.
4:20
List comprehension, denoted by the use
of square brackets, always produces
4:24
a list as its result, regardless of
the type of iterable used as the source.
4:30
As you can see with our banner example,
a string, when manipulated with
4:36
list comprehension, returns a list of
individual characters of that string.
4:40
There are many ways to convert
our list back to a string, but
4:47
the most efficient way of doing so here is
with the join method, let's break it down.
4:51
Iterable, is the iterable object that
contains values that we want to join
4:57
together to make a string.
5:01
For us, this is the return
value of a list comprehension.
5:04
Glue is a string that we want to use
to join our list values together.
5:10
I've called this glue because it's
what holds the string together.
5:15
In our case, we don't need any special
characters between our letters, so
5:19
we'll supply an empty string here.
5:24
So now that we know that,
5:28
let's use the join method to turn
this list back into a string.
5:29
We'll supply the glue, which is an empty
string, and then add the join syntax,
5:35
and surround our entire comprehension
syntax with wrapping brackets.
5:40
Let's make sure to save, and then we'll
run the file again by pressing Up and
5:46
Enter, and there we have it, nice work!
5:51
You've just learnt how to use the basic
list comprehension syntax with
5:55
two types of iterables, lists and strings.
6:00
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up