Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
Preview
Video Player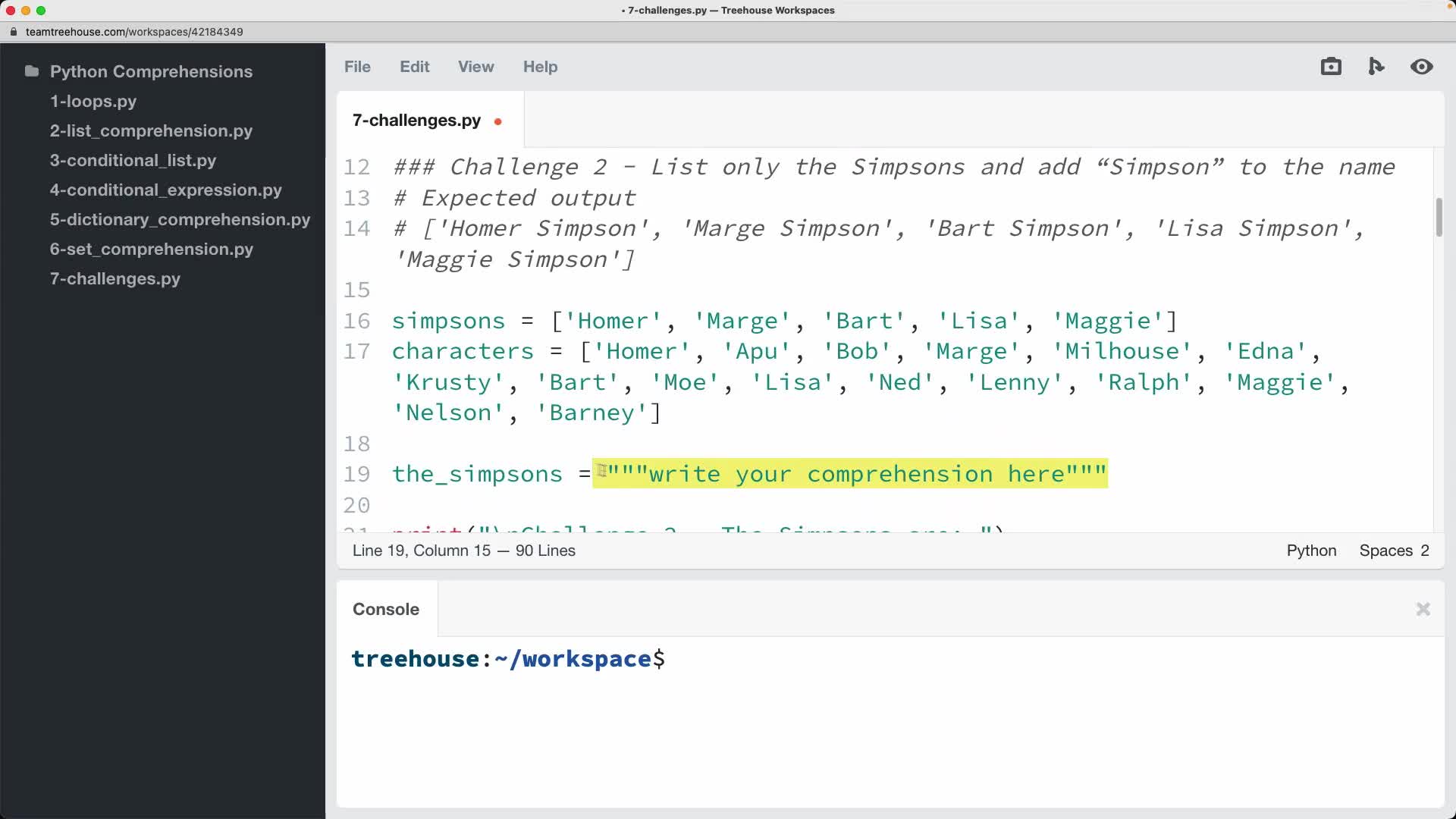
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
How did you go? In this video I’ll walk through what I did to complete these challenges.
Solutions
Challenge 1
leap_years = [year for year in range(1901, 2001) if year%4==0]
Challenge 2
the_simpsons = [f"{character} Simpson" for character in characters if character in simpsons]
Challenge 3
word_lengths = {word: len(word) for word in words if len(word)>=5}
Challenge 4
ages_next_year = {person: age+1 if person!='Danny' else age for (person, age) in ages.items()}
Challenge 5
vowels = {letter for letter in statement.lower() if letter in 'aeiou'}
Challenge 6
overlap = {food for food in fruits if food in vegetables}
Challenge 7
def format_name(honorific, candidate):
return honorific + " " + candidate.get('name').split()[-1]
graduates = [format_name("Professor", candidate) if candidate.get('score')>=90 else format_name("Doctor", candidate) for candidate in phd_candidates if candidate.get('score') >=70]
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
Welcome back!
0:00
I hope you had a good time
chewing on these challenges and
0:01
practicing your new skills.
0:04
Here are my solutions, but remember
that these are just my solutions, and
0:07
there could be a few different ones.
0:11
If yours differs to mine, don't worry, but
0:15
do feel free to share your
solutions in the forums!
0:17
I'll be walking through all the solutions
before running the file for the sake
0:21
of easy viewing, but feel free to be
running and testing your code as you go.
0:27
For the leap year challenge,
0:32
I started with square brackets
because I know I'm creating a list.
0:34
Now, my head works better if I
think about the loop syntax,
0:40
so I wrote that in first, for
year, in range, 1901 to 2001.
0:45
Then I'll pop in the expression,
which is just the year.
0:52
And then finally, the conditional.
0:57
If year%4=0.
0:59
Moving on to the Simpsons' list challenge.
1:05
Again, I started with square brackets
because I know I'm after a list,
1:09
and then a familiar loop syntax for
character in characters.
1:14
Then the expression.
1:21
Here I used an f string like so,
so f:{character},
1:23
Simpson to add the Simpson last name.
1:28
And then finally for the conditional,
if the character is in the simpsons list.
1:33
Now, moving on to challenge 3.
1:41
For this challenge,
we want to return a dictionary.
1:45
So we'll start with some curly brackets,
and
1:47
then the familiar loop syntax for
word in words.
1:51
Now since this is a dictionary,
the expression must be a key and value.
1:57
So in reference to the expected
output where there is a word and
2:02
then the word length, I used word for
the key and land word for the value.
2:07
Finally, the condition is if there
are five or more letters in the word.
2:14
So if len (word) is greater or equal to 5,
2:18
moving right along to challenge for
2:23
this challenge is also
often a dictionary output.
2:26
So we'll start with some curly brackets,
and then the loop syntax.
2:31
This time the original
iterable is a dictionary.
2:36
So I'll have to remember that there
are multiple temporary variables.
2:40
So we'll start with a template for
these in ages.
2:44
I'll also need to remember the items
method so that we can unpack the contents.
2:52
If we have a look at the original
dictionary, the key is a name, and
3:00
the value is the age.
3:05
So I'll call our temporary variables,
person and age.
3:08
For the expression,
I'll want to keep the person
3:15
as the key and then for
the value it will be age+1.
3:20
Now to handle Danny's case, I don't
want to remove Danny from the list.
3:25
So this won't be a list conditional.
3:30
So I'll add an if else statement in
the expression to test for Danny.
3:33
If person is not Danny,
if it is Danny, just the regular age.
3:38
And we'll pop back here because I realize
I missed the S to my items method.
3:45
On to challenge 5.
3:51
Since we're creating a set,
I'll start with the curly brackets.
3:53
As usual, I'll pop in the loop syntax for
letter and statement.
3:58
Now, I'm using letter because we're
gonna loop through every letter.
4:03
Now, I don't want to count
uppercase vowels and
4:07
lowercase vowels to be
different characters.
4:10
Otherwise we'll get this uppercase O and
4:12
all these lowercase o's
counted as unique letters.
4:15
Plus the challenge wants me to return
the set of vowels in lowercase.
4:19
So now is a great time to make the
statement lowercase with statement.lower.
4:23
For the expression,
I'll just need to specify letter.
4:29
And for the condition,
we're only interested if
4:34
the letter is a vowel, so
we'll use if letter 'aeiou'.
4:39
Now on to challenge 6.
4:44
Just like before,
I started with the curly brackets.
4:48
Now my plan for this challenge is to
loop through the fruits list, and
4:52
then check if that fruit is in
the vegetable list as well.
4:55
So, we'll start with, for food in fruits.
4:59
Now the expression just needs to return
the food as is, so it'll just be food.
5:04
And finally the condition is whether or
5:10
not the food is also in
the vegetable list as well.
5:12
So if food in vegetables.
5:16
And finally, challenge 7.
5:21
Give ourselves lots of room to work with.
5:25
Since we're after a list,
let's start with our square brackets.
5:29
The loop syntax I used here was for
5:34
candidate in phd_candidates.
5:38
For this challenge, it made sense to
tackle the list condition first for me.
5:42
So let's first cut any
candidate that scored below 70.
5:47
The candidate temporary variable
now holds a dictionary,
5:52
one of these every time, so that we
can access its values by using.get.
5:56
We want the score, so
our condition will be if
6:03
candidate.get score is greater or
equal to 70.
6:07
Now for our expression,
we'll also need a condition here,
6:13
depending on how they scored.
6:16
Here I used some placeholders
to get my logic in place first.
6:19
So my condition ended up being,
{"Professor"
6:23
if candidate.get('score') was greater or
6:29
equals to 90 else "Doctor".
6:34
Once that's in place,
6:39
I used f strings again to inject
the graduates name like sir.
6:41
Make that an f string, and
then {candidate.get('name')}.
6:47
And then the same over here for
Doctor{candidate.get('name')}.
6:55
But wait.
7:05
The instructions only wanted their
last name after the honorific.
7:05
So I handled that by splitting the name by
whitespace and grabbing the last element.
7:10
Split, and then the final element,
and split the final element.
7:17
Now this is looking a bit long, and
we're repeating ourselves a lot.
7:26
So let's write a helper function for
name formatting.
7:31
We'll call it format_name.
7:35
And it will take two parameters,
passing the honorific and
7:39
the candidate object,
which is an entire one of these.
7:43
For our function,
we will return the honorific plus
7:49
an empty space,
plus the candidate, get name.
7:54
And then we'll split it and
then grab the last element.
8:00
Come down here and we will replace
all of these with the format name.
8:05
The honorific here was Professor,
and we'll pass in the candidate.
8:12
And then we'll do the same here for
the Doctor one,
8:18
format name, honorific is Doctor,
and pass in the candidate.
8:22
Wow, that was a lot.
8:29
Now let's make sure we save our file.
8:31
And we'll make this as large as we can so
we can see everything.
8:34
And then let's run it.
8:37
Python3, 7, tab, Enter.
8:39
And we can see the print statements for
all of our challenges.
8:42
Well done.
8:47
Great job on those challenges.
8:50
No matter how you did on your own, know
that you've learned something new today,
8:52
and that you'll now be able to
add Python comprehensions to
8:57
your developer tool belt.
9:01
Well done.
9:03
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up