Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- Python Data Types and Math Operations
- Numbers 4 questions
- Booleans 7:51
- Logic 4 questions
- If, Else and Elif 11:12
- Use an if 1 objective
- Functions 11:37
- Reviewing Functions 3 questions
- Expecting Exceptions 7:15
- Exception Flow 2 questions
- While Loops 9:32
- Manners 2 questions
- The Random Library
- The Number Guessing Game
Preview
Video Player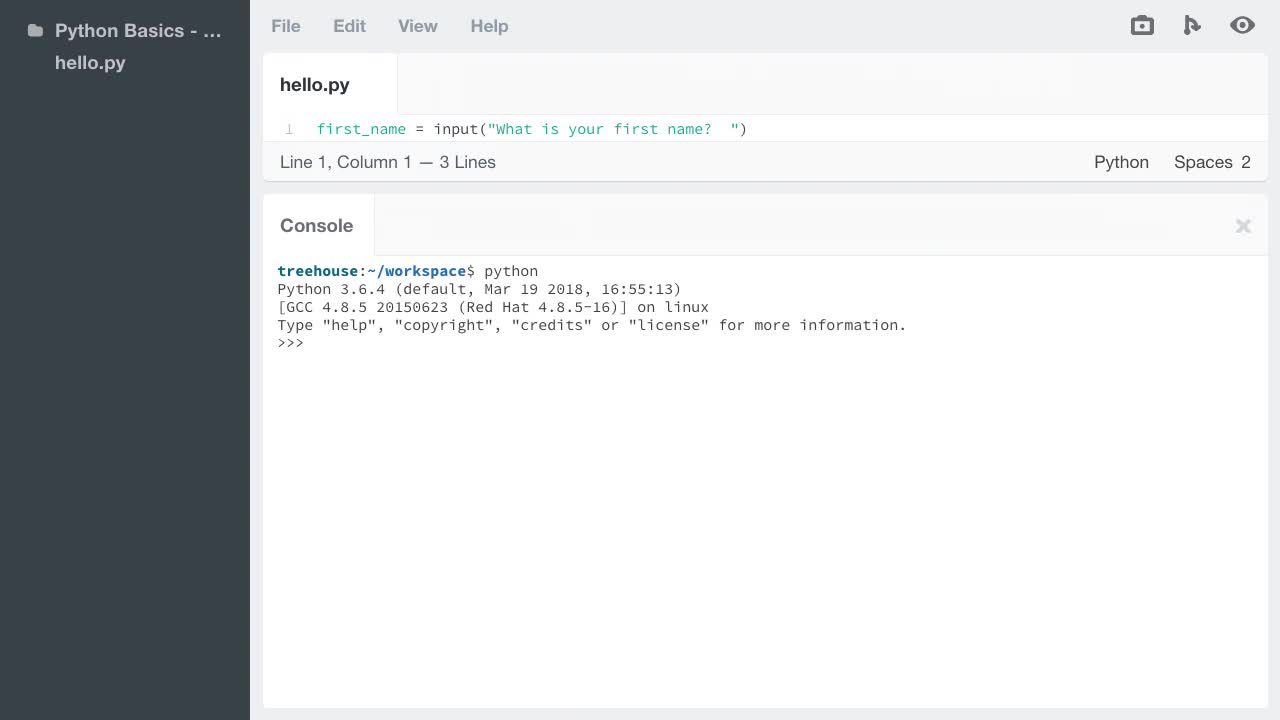
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Let's take a look at boolean literals True and False and how to use logic
This video doesn't have any notes.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
A Boolean data type only has two
valid values, true and false.
0:00
These values are going to be used
heavily in the code that we create from
0:05
here on out.
0:08
There are many times where we'll
want to ask a yes or no question and
0:09
then run different code based
on how the question's answered.
0:12
Booleans are named after their creator,
a mathematician named George Bool.
0:16
In George's algebra, he used ones
to represent the value true and
0:21
zeros to represent false.
0:25
And this is the reason you see
actors playing hackers and
0:27
movies dealing with ones and zeros.
0:30
There's a popular saying in this world
that says it's all ones and zeros, and
0:32
that's because it is.
0:36
Electrical circuits used in the computer
that you're using right now,
0:37
are using Boolean values on or
off, true or false, one or zero.
0:41
So they seem pretty important
to get a handle on, right?
0:45
We should explore these.
0:48
True or false?
0:49
The answer's true.
0:51
There are only two Boolean literals,
true and false.
0:54
A variable can refer to the Boolean result
in an expression like our n keyword,
0:58
for instance has_taco equals
is taco in catacombs.
1:05
You'll see that that was stored,
has_taco, you stored true in there.
1:11
And just like our other data types, you
can actually coerce values to be true or
1:16
false.
1:21
It's called bool.
1:22
And then, as you probably guessed,
1:23
bool (1) true, and
of course, bool (0) false.
1:27
Now here's something
that might surprise you,
1:32
in addition to being the answer to
the meaning of life, 42 is also true.
1:36
In fact, any non zero number is true and
zero is false.
1:42
So what happens with the string?
1:48
So if I say,
what's the Boolean of burrito?
1:50
Now I guess speaking
personally in the statement,
1:58
I want a burrito is always going to return
true, but that's not why this is true.
2:00
What's happening here is any
object that isn't empty is true.
2:04
Now, empty is an interesting concept
that we haven't talked about just yet.
2:09
So a string literal is a pair of
quotes surrounding a character, right?
2:13
But what if there aren't
any characters in there?
2:18
Now this is known as an empty string and
as you can see it's false.
2:22
This emptiness is false tends to
be a common confusion point for
2:28
developers, so
I want to introduce you to another term.
2:32
The way in which a value coerces
to a Boolean also has a name.
2:37
It's truthy, or falsey.
2:41
So for instance,
I can say that empty string is falsey, and
2:44
the number seven is truthy.
2:48
I know it sounds like I made that word up,
but I assure you it's real.
2:50
And you'll see it and hear it used.
2:54
Guess it's slightly better than
saying it's truish or falsey-ish.
2:56
So all that to say, emptiness is falsey.
3:01
That sounds like the name of an emo band.
3:05
I'd like to introduce you to a couple
of keywords that you already know and
3:09
use in real life.
3:13
You are about to see some of
the beauty of Python's readable syntax.
3:14
So if I wanted to negate a Boolean value,
get the opposite of the value.
3:18
I can use the not keyword, so
I can say, not true, and it says false.
3:24
Neat, right?
3:31
And if I say not false,
we'll see that that's actually true.
3:32
So next up, you can actually chain
together Booleans using the keyword,
3:35
and so if we say true and
true, we see that that's true.
3:43
The way that and works is that both
Booleans on both sides of the and
3:49
must be true.
3:53
So you can keep chaining them together
too, and it works just like order of
3:55
operations that are math examples
it goes from left to right.
3:59
So if I say true and true and
true, we get back true.
4:03
But what happened was it checked this
true and true, and that returned true.
4:10
So that true, the result of this was
checked against this true and true, and
4:15
both sides were true.
4:19
So, therefore it's true.
4:20
But if we come and
we slap a false at the very end and false,
4:22
we'll see that it's false because
all of this became true and
4:26
then we had true and false and
both sides must be true in order for and
4:30
to return true and
it wasn't so it returned false.
4:35
So ors work a little bit different.
4:38
If either value on either side of
the keyword or is true, it's true then.
4:41
Both ands work with both and
ors work with either.
4:48
So if it's false or true, we're gonna end
up with true because there's one true.
4:53
But if we have false or false, it's
gonna be false because nothing's true.
4:57
You can chain ors together too, and you
can also use parens just like we did with
5:04
our math to help it be more specific
about the order of what's happening.
5:08
So let's just go ahead,
let's build something random.
5:13
We'll say false or false or
5:16
true and true and false.
5:22
Okay, so let's walk through it
before we press Enter here.
5:28
So two falses ordered together,
that's a false.
5:30
And so we have a false or true.
5:34
One of those is true, so we have a true.
5:36
So we have true and true and
false, this is a false.
5:38
So we have true here and
we have false over here,
5:42
because not both of these are true.
5:44
So we have a false and
a true that should be false,
5:47
because both sides need to be true.
5:51
And remember, we can always negate.
5:55
We come in here, we can say and not.
5:59
Now you'll see that it looks
like I typed over the front.
6:03
This is a bug that sometimes
happens in the repo.
6:05
So I'm gonna press spacebar,
even though that looks wrong, watch.
6:08
Press Enter, it came true.
6:12
If we look at it, there it is.
6:14
So we have false or false or true and not.
6:15
And so that negated this last one here.
6:18
So this not true or false, true and
false was false, not false is true.
6:20
I know that this is all
a little bit abstract.
6:25
So let's try to make this
a little more concrete.
6:29
Let's think about a dating app and
6:32
let's try to imagine some
code that might exist there.
6:34
So Heidi has some kids.
6:39
She'd like to find someone who has
kids too, but she can't stand smoking.
6:42
She abhors it, so
let's fill out a profile for
6:46
a parent on the site who's also a smoker.
6:49
So is smoker equals true, and
6:53
they're a parent, so has kids equals true.
6:56
So Heidi's requirements look like this.
7:02
She says,
I want somebody who's a parent, so
7:04
he has kids and is not a smoker.
7:09
So we have in this particular
situation for her requirements.
7:12
This profile here, we have has kids.
7:17
That's true.
7:20
And not is smoker, so that's false.
7:21
So we have true and false.
7:24
That's a false, that didn't work out.
7:27
Heidi's gonna swipe left.
7:29
This just isn't the one for her, I guess.
7:30
Here's hoping that she finds her.
7:33
Do you see the power of Booleans?
7:34
They can help you to find true love.
7:37
Now, to really fall for Booleans, I'd like
to show off the ability to run different
7:40
blocks of code based on their value.
7:44
This is called conditional branching.
7:46
Let's branch off to that topic
right after this quick break
7:48
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up