Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- Python Data Types and Math Operations
- Numbers 4 questions
- Booleans 7:51
- Logic 4 questions
- If, Else and Elif 11:12
- Use an if 1 objective
- Functions 11:37
- Reviewing Functions 3 questions
- Expecting Exceptions 7:15
- Exception Flow 2 questions
- While Loops 9:32
- Manners 2 questions
- The Random Library
- The Number Guessing Game
Preview
Video Player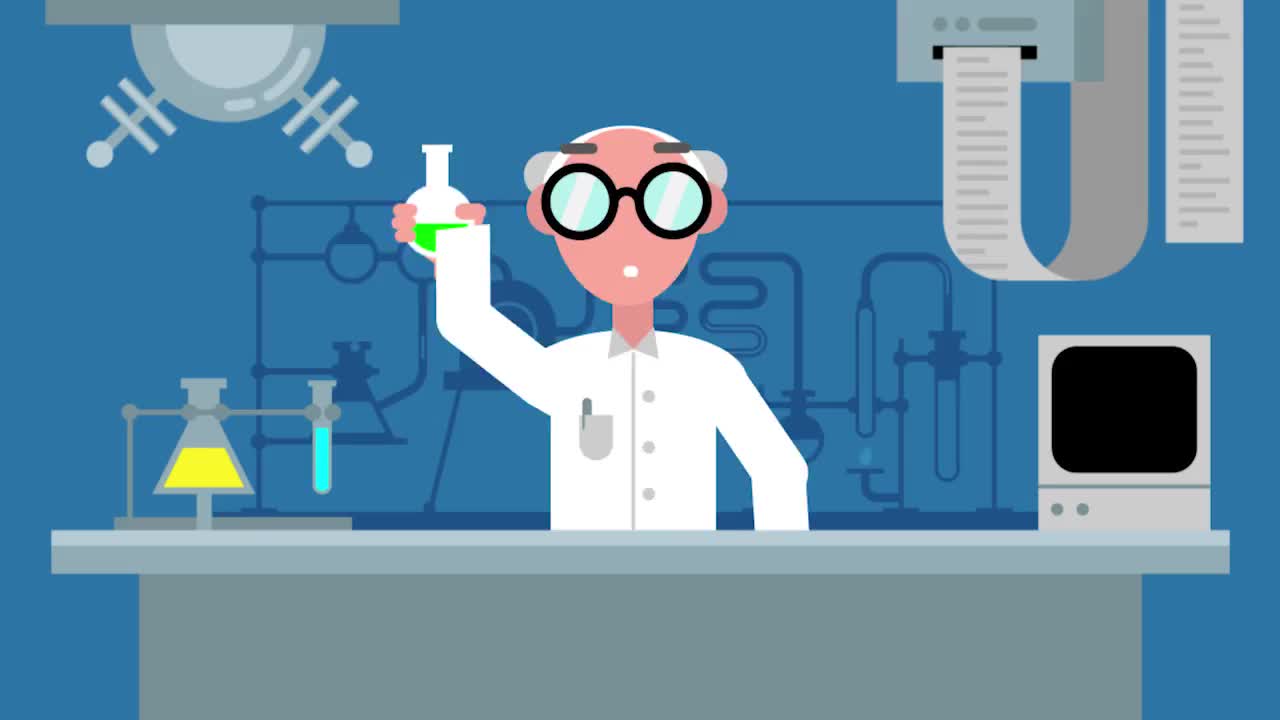
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Exceptional situations happen in our code and you can handle them in preparation. Let's learn how.
This video doesn't have any notes.
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
Murphy's Law states,
anything that can go wrong will go wrong.
0:01
Now, that's a pretty negative
way of looking at the world.
0:06
But it's a pretty good way to think about
how users might use your application.
0:09
If they can break it, they will.
0:13
We've seen some syntax errors getting
thrown when we write improper code.
0:16
But as we just witnessed,
0:20
our users can also create errors
as our program is running.
0:21
When they don't do what
we expected them to do,
0:25
these types of errors
are called exceptions.
0:27
The experience for a user,
0:31
when we run into one of these exceptions,
can be pretty intense.
0:32
There's a stack trace and
some of our code even, and a message and
0:35
our program didn't even finish.
0:38
It just stopped right there on
the line that caused the error.
0:40
We shouldn't show that to the user.
0:42
I'm sure you've seen an application where
the error wasn't handled properly..
0:44
The good news is that there is a way
to handle these exceptions gracefully.
0:49
Let's see if we can't clean up
those exceptions that we just saw.
0:53
So let's make our user facing
exception happen again.
0:56
Let's kick off the program.
0:59
So we'll say, python check_please.
1:00
And what was the total value?
1:06
It was too much.
1:08
Now obviously we can't coerce
that saying into a float.
1:11
So let's look what the error is though.
1:15
We definitely don't want
the user to see this right?
1:17
Can you imagine seeing this?
1:20
What is it traceback?
1:22
I just want to split my check,
so let's fix it.
1:23
If you have some code that you think might
cause for some exceptional activity like
1:26
any of this type coercion stuff, any of
that, it's great for this sort of thing.
1:31
You can put it in what is known as a try.
1:35
So try is a statement.
1:39
So let's do that, we'll say try and
of course, that's followed by a block.
1:40
So we need a colon.
1:46
And what you do is you put your possibly
problematic code in that try block.
1:47
So here let's move these in.
1:52
I'm just going to tab this in,
tab this in.
1:55
And now what we want to do is
write the code that handles
1:58
that this exception has happened.
2:02
So the keyword that we
want to use is except.
2:04
So we say except, now as you know,
there are different type of errors.
2:10
So you want to catch just this
one error that we are doing now.
2:15
And as we saw, it's a value error.
2:18
I'm gonna go ahead and I'm gonna right
click, copy and I wanna paste it here.
2:21
And then I wanna put a colon.
2:25
And now,
the body of this is what we'll run
2:29
should this specific exceptions happen.
2:32
So what do we want to say?
2:36
Well, let's keep it user friendly,
well say print, how about no,
2:38
that's not a valid value, try again.
2:44
Feel like I've seen that before, right?
2:50
All right, now let's see what we got.
2:52
Python check please.
2:57
What's the total?
2:59
Too much.
3:00
There's our message, no,
that's not a valid value please try again?
3:03
But then we got a traceback again.
3:06
Hmm, but look, this is different.
3:09
This is a name error.
3:11
And this says name total
due is not defined.
3:12
It's weird, it's right here.
3:16
Now in order to understand this,
3:18
we need to figure out what's
happening inside this try block.
3:20
So, we came in to the try block and
what happened was we asked for
3:23
this input and we returned a string and
then the float tried to coerce that.
3:27
And that raised an exception, it raised
a value ValueError exception, and
3:32
so what happened was this whole line,
never ran.
3:38
This float line ran, but it didn't
return because it hit the exception, so
3:41
it popped into here and it wrote this.
3:44
So what happened was this assignment never
actually occurred, it never happened.
3:46
So when we get down here,
we're talking about total_due, and
3:51
it was never assigned.
3:55
Aha, name error, what you talking about?
3:57
So what we really want is we
only want this code to run,
4:01
this amount due and actually
the print statement for that matter.
4:05
We only want that to run if and
only if there was no exceptions.
4:08
So the try block also allows for
what's known as an else.
4:14
Just like we saw in an if statement.
4:18
So let's do that.
4:19
So we'll say else.
4:20
And then that's a block and
we can bring these up.
4:22
So this can be read like try this
4:26
code, except if there is a value error.
4:31
In which case run this code.
4:36
Otherwise if everything's great,
run this code.
4:39
Now you might be thinking that I
definitely could have put this code
4:44
just up here in the try statement.
4:47
I didn't actually need this else.
4:49
But what I'm communicating to readers
of this particular code is that
4:51
this code here is expecting an error.
4:55
If I put this up here,
4:59
I'm not really worried about those
throwing a value error at the moment.
5:00
So this is specifically saying
these might throw a value error.
5:03
So what do you say?
5:09
Let's try it again, let's see how we did.
5:09
Check please.
5:11
Too much.
5:13
There we go.
5:16
No, that's not a valid value, try again.
5:17
Got it.
5:18
All right, so we now have our coercion
error handling working pretty smooth.
5:21
Let's see what happens
now with our zero error.
5:26
So again, we want python check_please.
5:29
What's the total?
5:33
It was 20 bucks and
there were zero people.
5:34
Okay, so now we still have
that zero division error.
5:37
So we could actually handle
multiple exception types.
5:42
So one thing that you could do
is you could just say accept
5:45
ZeroDivisionError and
then I'd write the code here, right?
5:50
Write some code here to do
write exception handling code.
5:58
But, wait a second, I'm gonna undo that.
6:02
So let's undo this, Cmd+Z again, undo.
6:06
I wanna note that the exception is coming
from within our split_check method.
6:12
I could wrap this math.ceil code
in a try and an except block.
6:18
But actually, if you think about it, zero
is an invalid value for this function.
6:21
You cant split a check with zero people.
6:28
Actually you know what else,
hold on a second.
6:31
What happens if we say
python check_please,
6:34
20 bucks, and we're going to split
that with negative 12 people.
6:38
Each person owes $-1, so does that
mean the restaurant owes us a dollar?
6:42
You can't split a check
with negative 12 people,
6:47
you can't even have negative 12 people.
6:49
So we really should consider less than or
equal to zero as an exception.
6:51
And we should raise that exception so
that others could catch it.
6:56
Just like this called a float did right?
6:59
Float said, hey I can't do that I'm gonna
raise an exception and then you handle it.
7:01
You know what we should do?
7:06
We should do that too.
7:07
We should make our split
check raise an exception.
7:07
It seems like good software design right?
7:10
Let's take a look at how to raise
exceptions right after this quick break.
7:12
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up