This workshop will be retired on May 1, 2025.
Heads up! To view this whole video, sign in with your Courses Plus account or enroll in your free 7-day trial. Sign In Enroll
Preview
Video Player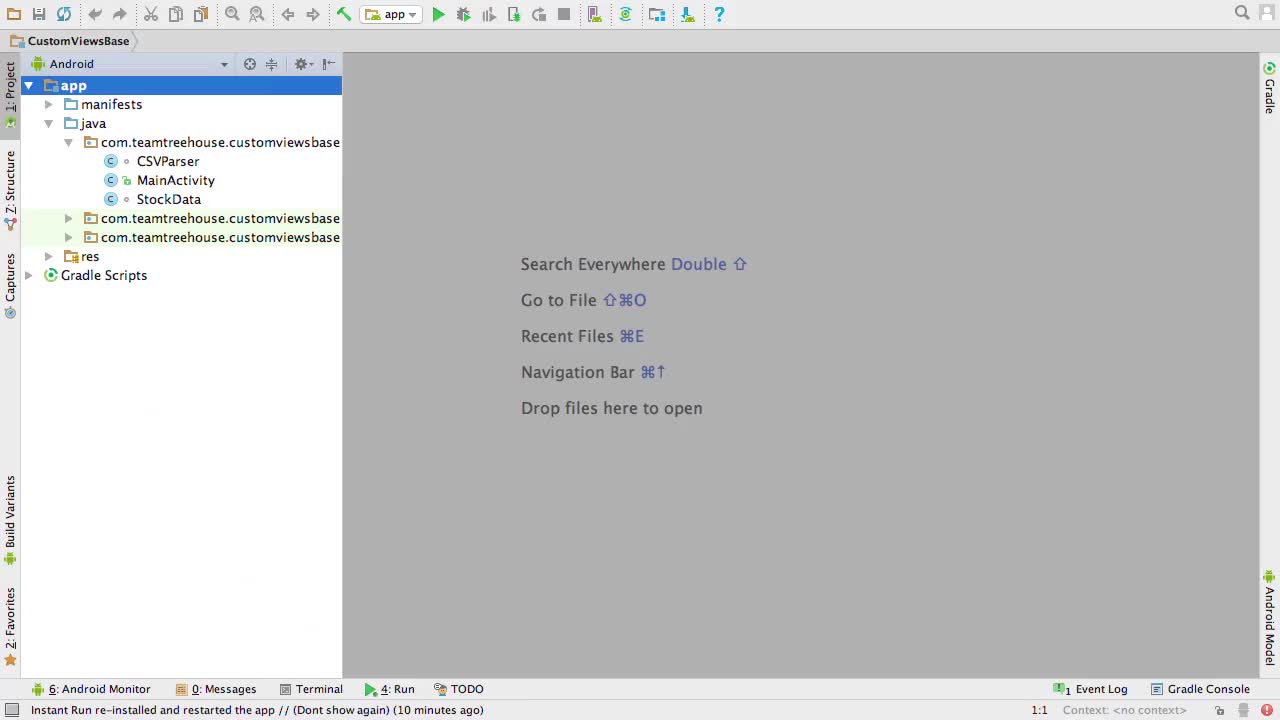
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
In this video we'll get acquainted with the app and learn how to use the Canvas object!
Related Links
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
Before we can use our custom view,
we're going to need an app to put it in.
0:00
So pause me and go ahead and
download it from the project files.
0:03
Cool, now that we've got the app,
let's open up MainActivity and
0:07
see how the app works.
0:10
We start by getting an input stream for
0:13
our CSV file,
which right now is named goog.csv.
0:15
And it exists inside our
resources inside the raw folder.
0:20
If you like to use your own data, just
paste the csv file into this folder and
0:25
then rename it to not
have any capital letters.
0:31
Then, open up your csv file and
delete the headers row.
0:33
Also, if your first row of data
has a time instead of a date,
0:38
delete that row as well, okay.
0:43
So back in MainActivity, we start by
getting our CSV file as an input stream.
0:45
Then we pass that input
stream to our CSV parser
0:50
which gives us back a list
of stock data objects.
0:55
And if we open up the stock data class,
it's just an object for
0:58
holding all the data from the CSV file.
1:02
And it also has a nice toString function.
1:04
Back in the onCreate method,
1:09
we loop through all the stock data and
print it out.
1:10
Seems simple enough.
1:14
Let's run the app and see what happens.
1:15
So we haven't really done
anything with the UI yet.
1:21
It's just one empty relative layout.
1:24
But if we check out the log,
We can see all of our data and
1:26
now we've just got to turn
this data into a custom view.
1:32
Let's start by creating
a new class named ChartView.
1:39
And let's make it extend
to the view class.
1:46
Then, let's use Alt+Enter to create
a constructor which takes in a context.
1:53
But before we get too far with our view,
2:00
let's make sure we know how
to add it to the screen.
2:02
Inside the constructor,
2:05
let's make our view stand out by adding
a call to setBackgroundColor and
2:06
passing in Color.RED.
2:12
Then, over in MainActivity,
let's delete the for loop.
2:17
And create a new chart view variable.
2:23
ChartView, which will call
ChartView equals new ChartView and
2:27
let's pass in this for the context.
2:31
Then let's create a relative layout
variable to store our root layout.
2:35
And let's call it relative layout and
set it equal to
2:42
casting to a relative layout
findViewById(R.id.).activity_main.
2:47
And finally, let's add our
ChartView to our relative layout,
2:57
relativeLayout.addview and
pass in our chartView.
3:00
Now if we run the app.
3:06
We get a completely red screen.
3:09
Awesome, okay, now that we've seen our
view, let's start turning it into a chart.
3:12
The first thing we'll need
to do is give it some data.
3:16
So let's press in our CSV resource as
the second parameter to our chart view.
3:19
And that's gonna be R.raw.goog.
3:27
At least for me.
3:29
Then lets use Alt+Enter to add
this parameter to the constructor.
3:31
Next, over in the trophy class,
let's create a new field to hold our data.
3:36
List, StockData and
we'll need to import list,
3:40
and let's name this data.
3:46
Then let's populate this
field in the constructor.
3:51
First, let's change the parameter
name from goog to resId.
3:54
Then let's cut out these two
lines from MainActivity,
3:58
And paste them into our
ChartView's constructor.
4:06
Finally, let's replace
R.raw..goog with resId.
4:11
And let's make this second line
refer to our new data field.
4:16
Nice.
4:23
Also, it looks like we've
got a warning on ChartView.
4:24
Custom view ChartView is missing
constructor used by tools.
4:27
If you want your custom view to play
nicely with Android's layout tools,
4:31
then you'll need to specify
one of these constructors.
4:35
We're not using the tools right now,
so we're fine ignoring this.
4:37
Next up is the onDraw function, which
is where we're going to draw our chart.
4:41
Let's add a couple lines
after our constructor, and
4:45
then use Ctrl+O to bring up the override
dialog, and then pick onDraw.
4:47
Which has a canvas object as parameter.
4:53
The canvas is just where
we'll be doing our drawing.
4:55
It has a certain width and height and
4:58
has a bunch of functions to help
us draw pretty much anything.
5:00
Also, we can get rid of this,
called a super.
5:03
Let's start this adventure by creating
a couple fields to hold the width and
5:05
height of the canvas.
5:08
Float width and height.
5:13
Then let's populate those fields and
the onDraw call,
5:18
width = canvas.getWidth and
height = canvas.getHeight.
5:24
Now that we've got that before we
try to do anything with our data.
5:30
Let's just try and draw a rectangle
on the bottom half of the screen.
5:34
On the next line,
let's type canvas.drawRect, and
5:37
then we need to pass in values for
each edge of our rectangle.
5:42
So let's pass in 0 for
the left edge, height / 2 for
5:46
the top, width for the right,
and 0 for the bottom.
5:52
Finally, we just need to
pass in a paint object.
5:58
So let's create a new paint field.
6:01
And let's name it paint and
set it equal to a new Paint object.
6:05
Then at the bottom of our constructor,
6:13
let's type paint.setColor and
pass in Color.Green.
6:17
Then let's pass on our new paint
object to our drawRect call.
6:23
And then let's run the app.
6:28
And now we've got a green rectangle
on the top half of the screen,
6:35
but we wanted it on the bottom half.
6:38
So what's going on here?
6:41
Well, an Android and a large part of
computers in general, the origin is
6:42
actually the upper left corner, and
the positive y direction is down.
6:47
So when we've got a top of
height divided by two and
6:53
a bottom of 0, we've actually
got our bottom above our top.
6:56
To fix this, let's change our bottom
from 0 to the height of the screen.
7:01
And if we run the app again, the bottom
of our screen is now a green rectangle.
7:08
That's enough learning
about the canvas for now.
7:14
In the next video,
we'll start drawing our chart.
7:16
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up