Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- What Will Be Built in This Stage 1:48
- Project Setup Using Vite 14:11
- Add Player Sprite and Movement 18:52
- Adding Gravity and Jump 12:16
- Create a Level Using Tiled 13:00
- Loading Tiled Level 11:39
- Animation and Level Design Quiz 5 questions
- Adding Collisions and a Background 8:04
- Adding Collectables 9:32
- Adding a HUD and Enemies 9:39
- Game Over Screen 12:03
- Adding Prefabs 13:45
- More on Prefabs 10:55
- Centralized Preloading 7:08
- Add an Exit and Another Level 11:53
- Music and Sound Effects 14:13
- Refactoring and Scene Management Quiz 5 questions
- Deployment and Game Improvements 10:07
- Game Development with Phaser Wrap-Up 1:38
Preview
Video Player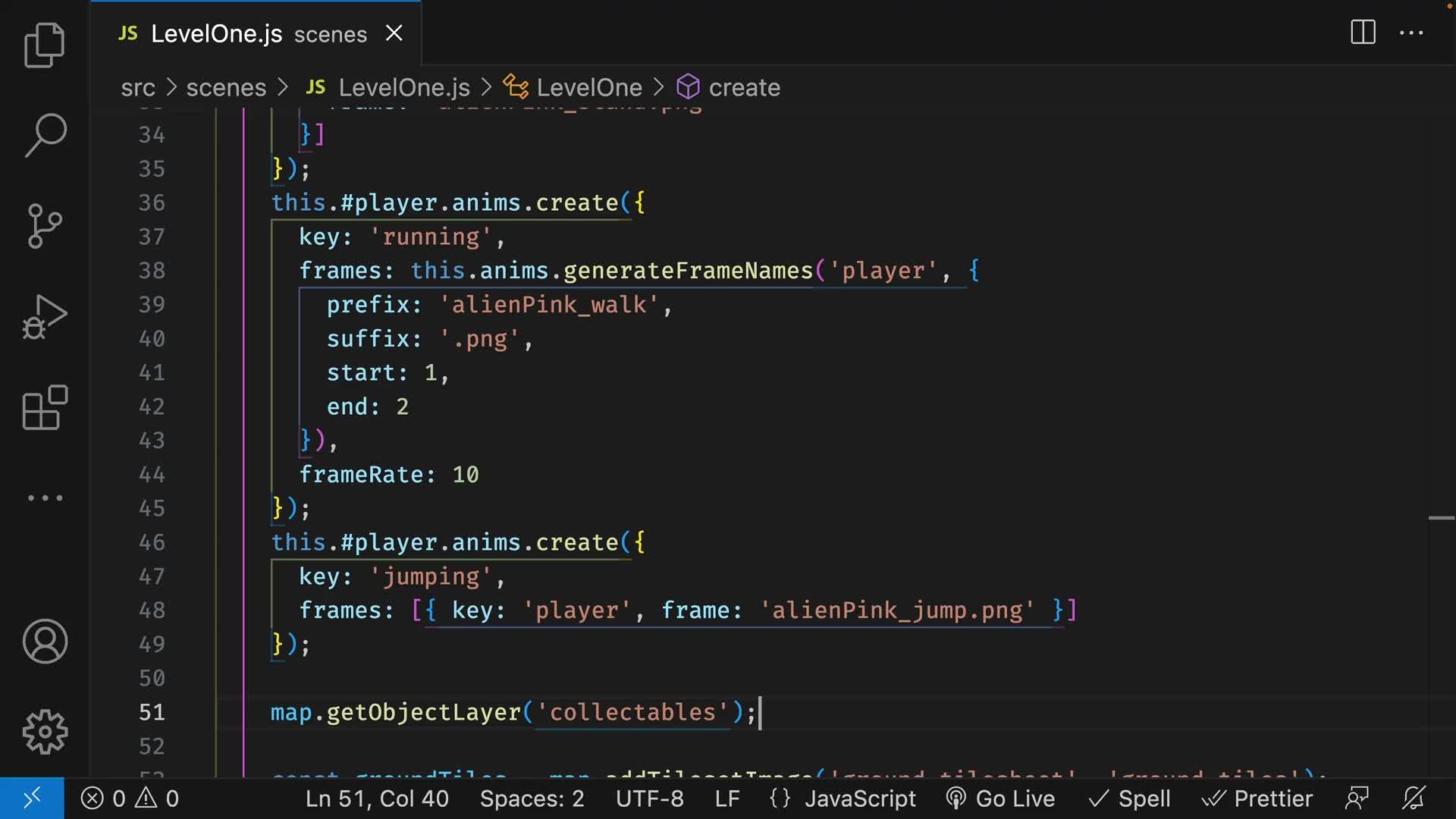
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Discover how to add collectables from Tiled and load them into our game.
Adjust Player's Collision box
// Add this to LevelOne.js to adjust the player’s collision box
this.#player.body.setSize(this.width, 150)
this.#player.setOffset(0,105)
Resources
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
In the last video, we went through how to
add collision detection to our level and
0:00
also how to add a background.
0:05
In this video, we're going to learn
how to add collectibles to our level.
0:07
If you remember from the previous videos,
we added some coins to our level in Tiled.
0:11
But we used an object layer for
0:17
our coins instead of a tile layer
which is what we used for our ground.
0:18
So the process to load the coins
in will be a little different.
0:24
Since we've already preloaded the coin
image in our preload method, we won't
0:29
have to do that again, we can just go
ahead and load in the layer from Tiled.
0:34
To do that, we're going to use
a method called getObjectLayer.
0:39
Let's scroll down to our create method.
0:43
And we'll go below where we've added
all our animations with a player.
0:46
At the end of line 49,
hit enter a few times and
0:50
write map.getObjectLayer
add parentheses and
0:54
inside the parentheses add
the string collectables.
0:59
The getObjectLayer method takes an
argument of a string which is the name of
1:03
the layer we want to load.
1:08
This needs to match the name
of the layer in Tiled.
1:10
Cool, now that we have access to all
the data in our collectibles layer,
1:14
what we want to do is loop over each
of the objects in the array to create
1:19
a sprite for each one.
1:24
In case you've forgotten what the objects
1:25
array looks like let's take a look
at it now in the level_1.json file.
1:28
You can see on line 29 that
this is the objects array for
1:34
the collectable layer, and you can see
how many objects we have in this array.
1:38
This is the array we want to loop over and
we want to use the X and
1:45
Y coordinates from each of the objects in
the array to position our coin sprite.
1:48
Let's do that now in
our LevelOne.js file.
1:54
Let's give our collectibles
objects layer a variable at
1:58
the beginning of life 51 right
const collectablesLayer equals.
2:02
Next let's create a group for
all the coins to go in.
2:06
This will save us from creating
a collider for each individual coin.
2:11
At the end of line 51, hit enter and
write const coins
2:16
equals this.physics.add.group and
add parentheses.
2:21
If we hover over the word group
we'll see that it's going to create
2:26
a dynamic physics objects for
each one of our coins.
2:30
A dynamic object is an object
that can move on its own or
2:34
can be moved by another
object through a collision.
2:38
In our case, our coins will not move and
they will not be moved by another object.
2:41
They're just going to be
collected by the player, so
2:47
we don't need them to be dynamic.
2:50
We can change them to a static
physics object instead.
2:52
Let's double-click on the word group and
change it to staticGroup.
2:56
Nice.
This means the objects will only be
3:01
updated once and not on every frame,
like a dynamic object.
3:04
Okay, let's go ahead and
3:10
loop over the objects array in
our collectibles layer variable.
3:11
At the end of line 52 hit
enter a few times and
3:16
write collectibles layer.objects.for
each and add parentheses.
3:19
Inside those parentheses let's
add another parentheses and
3:26
inside the second one
write the word object.
3:29
Then let's create an arrow function and
3:33
inside this function we want to
add a new coin to our coins group.
3:36
So we're going to write coins.create,
then add parentheses, and
3:41
inside the parentheses for the first
argument, we're going to write object.x.
3:46
For the second arguments,
we're going to write object.y.
3:52
And for the third argument,
we're going to put in a string of coin.
3:56
The create method is used to create a new
coin and add it to our coins group.
4:00
This takes in an argument of x and
y as the position, which we're getting
4:06
from our JSON file, and a key, which is
the name of the image we want to use.
4:11
And this should match the key of an image
that we set in our preload method.
4:16
Let's save this file and
take a look at our game in the browser.
4:21
Okay, so we can see that the coins
have been loaded, which is good, but
4:25
they're not quite in the right position.
4:29
This can happen sometimes.
4:32
The coordinate system in Tiled, when
it comes to objects, is different to
4:33
the coordinate system in Phaser.
4:37
This is really easy to fix.
4:39
First, let's go into our main.js file so
we can enable the physics debugger.
4:41
At the end of line 19,
hit enter and write debug: true.
4:47
Let's take a look at our
game in the browser again.
4:54
Okay, now we can see the bounding box for
each coin.
4:56
We can see that the coins
are positioned too low and
5:01
too far to the left from
where they should be.
5:04
Ideally, each coin should
be repositioned to
5:07
the top right corner
of their bounding box.
5:10
This helps with knowing where
to move the coin origin to.
5:14
You'll notice also the collision box for
the player is way above the player's head.
5:18
This is because the dimensions
of the player in the sprite
5:23
sheet.xml file is 128 by 256.
5:28
Our level in this course won't benefit
from adjusting the player's collision box.
5:31
But if it bothers you,
5:35
I've placed some code in the teacher's
notes that should fix it.
5:37
For now, let's focus on changing
the position of the coin.
5:41
Let's first give the coin object
in the loop a variable name.
5:46
At the beginning of line 55,
write const coin equals.
5:50
This should help with readability.
5:54
Next, let's add a set
origin method to the coin.
5:56
At the end of line 55, hit enter and
write coin.setOrigin.
6:00
Add parentheses, and inside the
parentheses give it a first argument of
6:05
zero and a second argument of one.
6:09
By default, the values in set
origin are both 0.5 this will
6:11
position the coin in
the center of the hitbox.
6:16
But by making the first value 0,
6:20
we're moving the x value
of the coin to the right.
6:22
And by making the second value 1, we're
moving the y value of the coin to the top.
6:25
Let's take a look at our
game in the browser.
6:31
Okay, we can see that the coins
are now in the right position.
6:33
But the hitbox for
each coin is still in the wrong position.
6:37
Let's change that now.
6:40
To fix that,
we can use a method called setOffset.
6:42
To match the new image position we want
the body to move half of the tile width
6:46
to the right, which is about 64 pixels and
half of the tile width to the top.
6:51
So let's write the code for that.
6:57
At the end of line 56, hit enter and
write coin.setOffset,
6:59
add parentheses, and
inside the parentheses,
7:03
let's give it a first argument
of object.width divided by 2.
7:07
Let's give it a second argument of
minus object.width divided by 2.
7:13
This is going to move the hitbox half
of the tile width to the right and
7:19
half of the tile width to the top.
7:23
And that should be it.
7:26
Let's take a look at
the game in the browser.
7:27
Nice.
7:29
We should see that the coins
are now in the right position.
7:30
But if the player walks over them,
nothing happens.
7:34
Let's add some code to the coins to
make them disappear when the player
7:37
overlaps them.
7:41
Before we do that,
let's go back to our main.js file and
7:43
remove the physics debugger
since we no longer need it.
7:46
Now let's go back to our LevelOne.js file.
7:50
We've used the physics.collider method
a few times in this course, but for
7:53
this interaction we're going to use
a physics method called overlap.
7:58
The Overlap method is very
similar to the Collider method.
8:03
In fact if we used Collider instead of
Overlap for this, it should work fine.
8:07
However, since the Overlap method
knows it's not going to be dealing
8:13
with collisions,
8:17
it doesn't need to carry as much
information as the Collider method does.
8:18
This will help our game with
its overall performance.
8:22
Okay, let's scroll down to
the end of line 68 and hit enter,
8:26
then write this.physics.add.overlap
add parentheses and
8:31
inside the parentheses give
it a first argument of
8:36
this.#player, a second
argument of coins.
8:40
And the third argument is going
to be a callback function.
8:45
So, let's give it some parentheses.
8:48
And we will give the second
parenthesis 2 arguments.
8:50
The first of underscore player, since we
are not going to use that player variable,
8:53
we give it an underscore, and
a second argument of coin.
8:57
Then, let's open up the callback function.
9:01
And online 70 we're gonna write
coin.destroy and add parentheses.
9:03
Nice.
9:09
Let's see what this does in the browser.
9:09
Okay, now if we hold on to
the right key and overlap a coin,
9:12
you can see it disappears.
9:16
Very cool.
9:18
This game is coming along nicely.
9:19
In the next video,
we're going to add a score to our game.
9:21
And whenever we overlap a coin we're going
to increment the score to show the total
9:25
value of the coins collected.
9:29
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up