Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- What Will Be Built in This Stage 1:48
- Project Setup Using Vite 14:11
- Add Player Sprite and Movement 18:52
- Adding Gravity and Jump 12:16
- Create a Level Using Tiled 13:00
- Loading Tiled Level 11:39
- Animation and Level Design Quiz 5 questions
- Adding Collisions and a Background 8:04
- Adding Collectables 9:32
- Adding a HUD and Enemies 9:39
- Game Over Screen 12:03
- Adding Prefabs 13:45
- More on Prefabs 10:55
- Centralized Preloading 7:08
- Add an Exit and Another Level 11:53
- Music and Sound Effects 14:13
- Refactoring and Scene Management Quiz 5 questions
- Deployment and Game Improvements 10:07
- Game Development with Phaser Wrap-Up 1:38
Preview
Video Player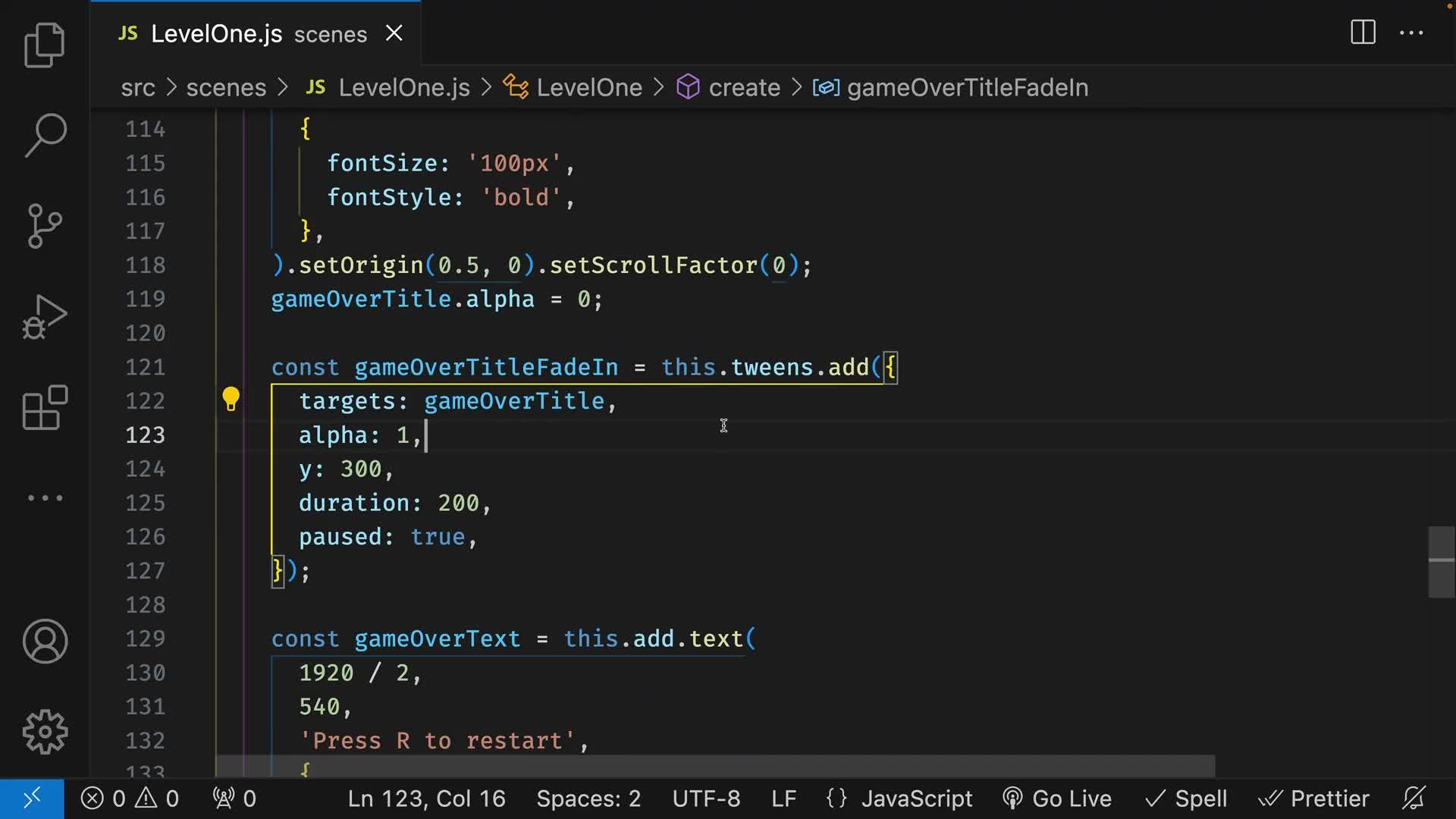
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Learn how to organize your code into modular components called prefabs, that can be easily reused and maintained, improving overall code structure of our game.
Solution code:
const gameOverTitle = this.add.text(
1920 / 2,
840,
'GAME OVER',
{
fontSize: '100px',
fontStyle: 'bold',
},
).setOrigin(0.5, 0).setScrollFactor(0);
gameOverTitle.alpha = 0;
const gameOverTitleFadeIn = this.tweens.add({
targets: gameOverTitle,
alpha: 1,
y: 300,
duration: 200,
paused: true,
});
const gameOverText = this.add.text(
1920 / 2,
540,
'Press R to restart',
{
fontSize: '50px',
fontStyle: 'bold',
},
).setOrigin(0.5, 0).setScrollFactor(0);
gameOverText.alpha = 0;
const gameOverTextFadeIn = this.tweens.add({
targets: gameOverText,
alpha: 1,
duration: 200,
delay: 200,
paused: true,
});
Resources
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
In the last video,
0:00
we triggered a game over screen when
the player overlaps with an enemy.
0:01
We did everything apart
from adding the text, so
0:05
we'll go through that in this video.
0:08
We're also going to refactor our code
by breaking things up into prefabs.
0:11
But for now,
let's go through the game over text.
0:15
If you didn't attempt to add
the text yourself, don't worry,
0:18
I've added my solution to the notes
tab at the bottom of this video.
0:22
Okay, let's scroll down to
the bottom of the create method, and
0:26
you'll see the new code that
I've added looks very familiar.
0:31
Let's quickly go over it.
0:38
On line 110, I've added a new variable
called gameOverTitle and this is
0:40
the text for Game Over that will show
when the player interacts with an enemy.
0:46
You can see it contains a string of
Game Over, and it has a font size of
0:51
100 pixels with a font style of bold,
and it also has a set origin and
0:55
a scroll factor similar to what we've
done before, as well as an alpha.
1:00
I've then created
a gameOverTitleFadeIn variable on line
1:05
121 that will fade in
the gameOverTitle on the y-axis and
1:10
the alpha similar to the background image.
1:14
Then I've created some more
gameOverText on line 129 and
1:18
this says press R to restart.
1:22
This is slightly smaller than the other
gameOverText, add a font size of 50,
1:24
but has a similar set origin and
scroll factor and alpha.
1:29
We're going to animate this text as well,
but on line 144,
1:33
it has a delay of 200,
which means it will animate 200
1:38
milliseconds after
the main game over text.
1:43
Finally, on line 154 and 155,
I've added the code to play
1:46
these animations when the player
interacts with an enemy.
1:51
Cool.
1:55
Let's see what this looks
like in the browser.
1:56
Okay, I'm going to move the character by
pressing the right key on the keyboard,
1:59
jump onto a platform and
then onto the enemy.
2:04
And you can see the background
darkens the game over image shows and
2:07
I get text that says game over and
later press R to restart animates in.
2:11
Nice, this is looking good.
2:17
But there's one small problem whenever I
press the R key on my keyboard to restart
2:19
the game, nothing happens,
that's because we haven't yet
2:24
implemented the restart logic.
2:28
Let's do that now.
2:30
Let's first make sure we're capturing the
R key when it's pressed on the keyboard.
2:32
Let's scroll to the top of the file and
below isGameOver text,
2:37
we'll create a new private class field
called RKey, then let's give it a type.
2:42
At the end of line 10 hit enter
right slash add two stars and
2:49
press ENTER and on line 12 right
at type add curly braces and
2:54
inside the curly braces, we're going
to write {Phaser.Input.Keyboard.Key}.
2:59
This should now give us auto complete
options for phaser keyboard objects.
3:07
Now let's create a key object, but
3:12
this time we're going to do it in
a method called init instead of create.
3:14
At the end of line 28,
hit enter a few times and write init,
3:19
add parentheses,
then add curly braces and hit enter.
3:24
And on line 31, write this.#RKey =
3:28
this.input.keyboard.addKey add () and
3:33
inside the parenthesis add a string of R.
3:39
This could have been put
in the create method, but
3:44
it's not going to be
displayed on the screen.
3:47
We could have also put this
in the preload method, but
3:50
the scene manager checks that all assets
are loaded in the preload method,
3:53
before moving on to the create method.
3:58
We don't need to check that the R key
is loaded before moving on to create.
4:00
So there's no need to put
it in the preload method.
4:05
Putting it in the init method makes more
sense, since the scene manager doesn't
4:09
check that the init method is complete
before moving on to the create method.
4:14
Now let's scroll down
to our update method,
4:19
and inside our guard clause on line 171.
4:24
We can add some logic to restart
the game when the R key is pressed.
4:28
At the end of line 171, hit Enter and
write if, add parenthesis and
4:33
inside the parenthesis,
write this.#RKey.isDown,
4:39
then our curly braces at
the end of line 172, hit Enter.
4:44
And at the beginning of line 173 write,
4:50
this.#isGameOver = false,
then on line 174,
4:54
write this.scene.restart and add ().
5:00
In the previous stage,
5:04
I suggested using the LocationReload
method to restart the scene.
5:06
This was okay since there was only
one scene in our game, so reloading
5:10
the whole page was fine, but for this
game we're going to have multiple levels.
5:14
So, if we were on level 2 and reloaded the
whole game, we'd be taken back to level 1.
5:19
With the scene restart method,
this only reloads the current scene and
5:26
not the whole game.
5:31
Because of that, we have to manually set
the isGameOver class field property
5:32
to false, or if we came back to the scene,
the isGameOver property would be true.
5:38
Okay, let's test this in the browser.
5:45
Again, let's press the right
key to move in the game.
5:48
Jump on top of the platform and
interact with an enemy.
5:51
Now, we can see the game over text.
5:54
Let's press the R button, and
we can see the scene restarts, and
5:57
we're back to the start of the level.
6:01
Perfect.
6:03
Let's go back to our code.
6:04
Right now, our level 1 file contains
a lot of code for the level.
6:05
If we wanted to make a level 2 file,
we need to copy all the code for
6:11
the player including the animations,
the code for the collectibles and
6:15
enemies all into that level two file,
and that is unnecessary duplication.
6:20
If you're familiar with JavaScript
frameworks like React, there's a concept
6:25
of components where you can break
code up into smaller reusable pieces.
6:30
When it comes to game development,
we can use something called prefabs and
6:34
although these aren't exactly
the same as components,
6:39
they allow us to break our code
into smaller reusable pieces.
6:42
The name prefab stands for prefabricated,
6:46
and it refers to a pre-made
game object that can be reused.
6:50
We'll start by creating a prefab for
the player.
6:54
Let's open up the File Explorer and
6:58
create a new folder in the source
folder called prefabs.
7:00
Then, inside the prefabs folder,
7:05
we're going to create a new
file called Player.js.
7:07
In this file, we're going to
create a new class called Player.
7:11
On line one,
let's write export default class Player
7:15
extends Phaser.Physics.Arcade.Sprite,
7:22
then add curly braces.
7:27
The player class extends
the Phaser.Physics.Arcade.Sprite class,
7:30
because the player is essentially
a sprite with physics.
7:35
This will give our class
access to all the methods and
7:38
properties that belong to
a phaser sprite class.
7:41
In our player class, we're going
to create a constructor method.
7:45
The constructor method is what runs when
a new instance of a class is created.
7:48
A constructor can take arguments
that are passed into the class.
7:53
So, let's create a constructor
with a few arguments.
7:56
At the beginning of line 2, write
the word constructor, add parentheses and
8:00
inside the parentheses we're
going to give it some arguments.
8:05
A first argument called scene.
8:09
A second argument called x,
a third argument of y and
8:11
a fourth argument of texture,
then add curly braces.
8:15
The scene argument is the scene that
we want the player to be used in.
8:19
x and y are the coordinates of where
we want to place the player, and
8:23
the texture is the name of the image or
asset we want to use for the player.
8:27
In the constructor method,
we're going to go something called Super.
8:32
The super method is something that will
call the constructor of the class we're
8:36
extending.
8:40
So if we option click on the sprite class,
8:41
we can see that it has its own constructor
method, which takes in the same
8:44
arguments that we're taking in for
our player constructor.
8:49
It's the same we can't see the code
implementation of the sprite class.
8:53
But the super method allows
us to use all the methods and
8:57
properties that are defined
in this constructor.
9:01
Let's go back to our player class and
call the super method as it entered in
9:04
between the curly braces, then on
line three, write super, parenthesis.
9:09
And inside the parentheses write Scene,
x, y, texture.
9:14
Nice!
9:20
Now let's add some code to our
player class from our level 1 class.
9:21
In our create method,
9:26
let's select all the code from line
40 all the way down to line 61.
9:28
This contains all the code for our player.
9:34
We're gonna press Command X
to cut this code, and
9:36
we'll go back to our player.js file,
and at the end of line 3 hit Enter,
9:40
and we're going to press Command V
to paste in all this code.
9:45
Since this keyword in our player
class refers to the player class and
9:50
not the faces' scene anymore, we can get
rid of all the references to #Player.
9:54
Nice.
10:00
Above the setCollideWorldBounds method,
10:02
we need to add the player to the scene and
also the scene physics to the player.
10:05
To do that, go to line 4, hit Enter,
10:10
write scene.physics.add.existing
at parentheses,
10:14
and inside the parentheses,
add a value of this.
10:19
Then hit Enter, and on line 6,
write scene.add.existing (),
10:23
and also in the parentheses,
add a value of this.
10:30
Cool.
10:35
We're not quite done yet.
10:36
Let's add the player movement
to this class as well.
10:37
We're going to create a new method
on line 32, called update () {}.
10:40
And let's go back to our level 1
file to get all the necessary code.
10:48
We'll start off by getting all the code
that's needed to move the player with
10:52
the keyboard.
10:56
Select all the code on line 36 and
press Command X to cut.
10:57
And we'll paste it at the end of our
constructor in our player class,
11:02
on line 31.
11:07
Next, let's scroll to
the top of this file.
11:08
Select our keys private field online 5 and
select the types related to the keys
11:11
field, press command x, and
paste it at the top of the player class.
11:16
Let's go back to our level1.js file, and
11:20
now let's scroll down
to the update methods.
11:23
And select the first two lines, that's the
destructured line on 144 with the left,
11:28
right, up and space variables and
also the movement anim variable.
11:35
We'll press command X to cut these lines,
and
11:40
we'll paste them in the update
method of the player class.
11:42
Make sure to remove
the reference to #player.
11:46
Next, we'll go back to the level 1
class and inside the update method,
11:49
select the rest of
the player movement code, so
11:54
everything from 154 to 165,
press Command X to cut that code.
11:57
Now let's go back to the player class.
12:01
Let's paste that code
underneath the movement and
12:04
in variable inside our update
method in our player class.
12:07
Again, make sure to remove all
the references to #player.
12:10
Cool.
12:14
And just like that,
we've created a prefab for our player.
12:15
Now, in our level 1 file, all we have
to do is scroll up to the top, and
12:19
find our reference to our hashtag player
class field and make sure we update
12:24
it to use our new player prefab instead
of adding a sprite to the faces' scene.
12:29
Cool.
12:35
Because of the NPM IntelliSense plugin,
12:36
our prefab class should be
automatically imported.
12:38
Make sure you scroll to
the top of the file and
12:42
check the import statement is there.
12:44
If not, you can add it manually.
12:47
I almost forgot, we have to scroll
down to our new player class and
12:49
add an argument at the front called this,
which refers to this scene.
12:53
Then, let's scroll down
to our update method and
12:57
run the update method
from our player class.
13:01
To do that online 147, right,
this.#player.update (),
13:04
this should enable player movement.
13:10
Finally, inside our player class,
make sure we're using the word scene
13:14
instead of this, where we have
our key's private class field.
13:18
Nice!
13:23
Let's test if this works in the browser.
13:23
And if all has gone well,
our game should work as normal.
13:26
And if I press the right key to move
the player, the movement works fine,
13:29
I can jump, I can collect coins and
I can also interact with enemies.
13:33
Cool!
13:36
This is a great start, but
we still have some more refactoring to do.
13:37
In the next video, we're going to
make a few more prefabs for our game.
13:41
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up