Heads up! To view this whole video, sign in with your Courses account or enroll in your free 7-day trial. Sign In Enroll
- What Will Be Built in This Stage 1:48
- Project Setup Using Vite 14:11
- Add Player Sprite and Movement 18:52
- Adding Gravity and Jump 12:16
- Create a Level Using Tiled 13:00
- Loading Tiled Level 11:39
- Animation and Level Design Quiz 5 questions
- Adding Collisions and a Background 8:04
- Adding Collectables 9:32
- Adding a HUD and Enemies 9:39
- Game Over Screen 12:03
- Adding Prefabs 13:45
- More on Prefabs 10:55
- Centralized Preloading 7:08
- Add an Exit and Another Level 11:53
- Music and Sound Effects 14:13
- Refactoring and Scene Management Quiz 5 questions
- Deployment and Game Improvements 10:07
- Game Development with Phaser Wrap-Up 1:38
Preview
Video Player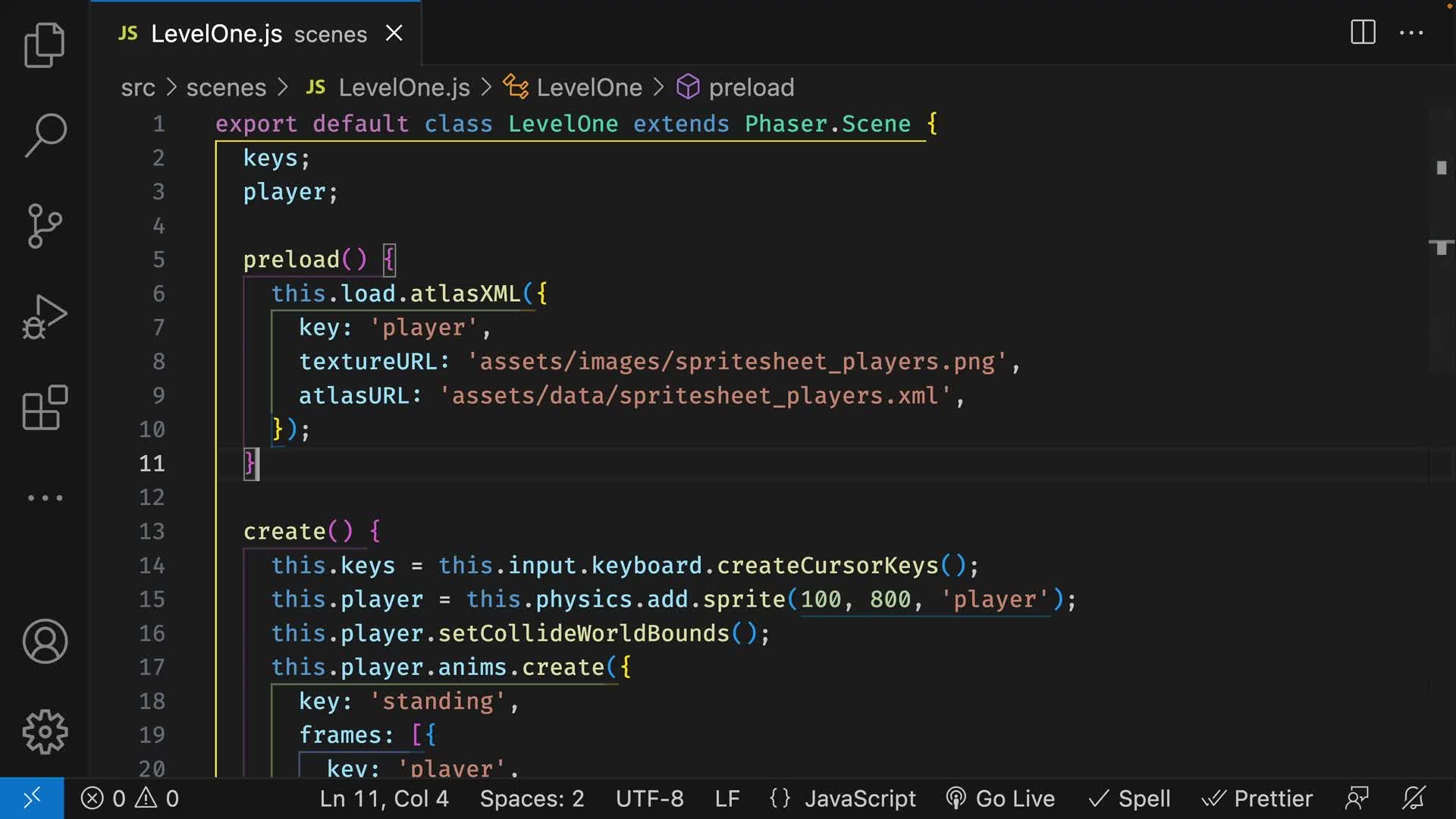
00:00
00:00
00:00
- 2x 2x
- 1.75x 1.75x
- 1.5x 1.5x
- 1.25x 1.25x
- 1.1x 1.1x
- 1x 1x
- 0.75x 0.75x
- 0.5x 0.5x
Understand how to use gravity provided by the Arcade physics engine to allow our player to jump.
Resources
- jsDoc
- Private class features
- Phaser Docs
Related Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign upRelated Discussions
Have questions about this video? Start a discussion with the community and Treehouse staff.
Sign up
In this video,
we're going to add some gravity and
0:00
give our character the ability to jump.
0:03
But before we do that,
let's refactor the code we have.
0:05
There are a few potential
issues with the code right now.
0:08
For example, if we were to come back
to this code in a few months or
0:11
maybe years time without using Phaser, and
0:15
look at some of the lines of codes in
the create or even the update method.
0:17
It would be difficult to tell
at a glance which methods and
0:22
properties were created by us and
which ones belong to Phaser.
0:25
For example, on line 14,
we know that this.input comes from Phaser,
0:30
and on line 16 that
this.player comes from us.
0:36
And we know that because we can look
at the top at lines 2 and 3 and
0:41
see that we've created these class fields.
0:45
However, without that prior knowledge,
0:48
it's difficult to tell which
method belongs to who.
0:51
We can make our custom
fields more obvious.
0:54
We can give them something called a hash
name, turning them into a private field.
0:57
Private fields make it so that these
fields cannot be accessed externally
1:03
when the class has been instantiated.
1:07
Because of the way
the Phaser scene is set up,
1:10
we won't truly benefit from all
the advantages a private field gives us.
1:13
But this will help us easily differentiate
between the Phaser properties and
1:17
our own fields.
1:23
At the beginning of line 2,
let's add a hashtag or
1:24
pound sign before our keys custom field,
and
1:27
let's do the same at the beginning of
line 3 for our player custom field.
1:30
Next, let's select the first occurrence of
this.keys in our code, this is on line 14.
1:35
Then press Shift+Cmd+L to select
all the other occurrences,
1:42
and add a hashtag before the letter k.
1:49
Cool, let's do the same with
our player class field.
1:52
Select the first occurrence of this.player
on line 15, then press Shift+Cmd+L to
1:56
select all the other occurrences, then add
a hashtag before the letter p on player.
2:02
Now, when we look at the create or
update methods, it's super obvious
2:07
which properties are coming from Phaser
and which ones were created by us.
2:12
While we're in the update function,
2:17
let's do some refactoring to
reduce the amount of duplication.
2:19
First, let's destructure the left and
2:23
right variables from this.keys so
it's easier to read.
2:26
At the end of line 36, hit Enter and
write consts, then add curly braces.
2:30
And inside the curly braces, write left,
2:36
right, and then add = this.#keys.
2:41
We can now update the code accordingly by
2:46
deleting the occurrences of
this.keys in the update method.
2:48
Select the first occurrence of
this.keys on line 38, then press Cmd+D
2:53
to select the next occurrence,
then press Backspace to delete it.
2:58
Next, let's reduce the amount of
blocks we have in our if statement by
3:03
using some ternaries
inside the first if block.
3:08
Let's combine the left.isDown and
the right.isDown in the first block
3:11
by adding a pipe at the end of left.isDown
on line 38, then adding right.isDown.
3:17
After that, on line 39,
in the parentheses for
3:23
the setVelocityX method, write
left.isDown, then add a question mark,
3:27
then after -400, add a colon,
and then add 400.
3:33
This makes sure that the player
velocity changes based on if the left
3:37
button is being pressed.
3:42
Next, on line 40, in the parentheses for
3:43
the setFlipX method,
change true to left.isDown.
3:47
This means that if the left button is
being pressed, the player will be flipped,
3:52
and if the right button is pressed,
then the player won't be flipped.
3:56
Since the rest of the code is
similar in the else if block,
4:01
we can highlight all the code from
line 45 to line 42 and delete it.
4:05
Nice, our update function
is now a lot cleaner.
4:10
Let's do one more thing before
we move on to adding gravity.
4:14
You'll notice that if we hover over any
methods used by our custom class fields,
4:17
we get an any.
4:23
This is because no type
information has been set for them.
4:24
So VS Code doesn't know what these are.
4:28
Even though we're not using TypeScript, we
can still set types to our custom fields,
4:32
and this can be done through
something called JSDoc.
4:37
JSDoc allows us to add multiple things
to our variables, but in this case,
4:40
we can add types to them using comments,
and
4:45
these types will be applied wherever
these class fields are used.
4:48
If you want to learn more about JSDoc,
4:52
I'll add a link to the documentation
in the teacher's notes below.
4:54
Let's go to the top of our file,
And at the beginning of line 2,
4:58
hit Enter, and write /,
add two stars, and then hit Enter.
5:05
VS Code should add the remaining star and
slash for you.
5:10
Then write @type and
then add curly braces.
5:13
And inside the curly braces,
5:17
type
Phaser.Types.Input.Keyboard.CursorKeys.
5:21
This is because we want our keys field to
5:27
have the same type as
the create CursorKeys method.
5:30
I worked out these types
before recording the course by
5:33
not assigning any custom class field
to the create CursorKeys method and
5:37
seeing what type Phaser
gives it by default.
5:41
You can use the same technique if you
want to add types to class fields for
5:44
future games you want to make in Phaser.
5:48
Now, if we scroll down to our update
method and hover over left, all right,
5:50
on line 41, we get some information
about these methods and properties.
5:55
Let's do the same to our player field.
6:00
Let's scroll up to the top of the class,
and
6:03
at the end of line 5, hit Enter,
write slash and add two stars.
6:07
Press Enter again and write @type,
add curly braces and write
6:12
Phaser.Types.Physics.Arcade.SpriteWithDyn-
amicBody.
6:18
Sweet, again,
if we scroll down to the update method and
6:24
hover over any property
used by our player field,
6:28
we can see we get some
useful information about it.
6:31
Cool, let's now add some
gravity to our game.
6:35
We can do this quite easily
in the main.js file.
6:38
To get to that file,
type Cmd+P on your keyboard, and
6:42
in the search box, type main and
select main.js from the drop-down.
6:46
Right now, in the config object,
gravity on line 17 is set to false.
6:51
But because we want to add gravity,
6:56
let's change that to an empty object,
and inside, write y : 800.
6:58
This adds an acceleration of
800 pixels on the y-axis of any
7:04
physics body in our game.
7:09
Let's see how this has changed
our game in the browser.
7:11
You can now see that when we start our
game, our player falls to the ground.
7:15
And because if the set
collider world bounds,
7:19
the player doesn't fall below
the lower screen boundary.
7:22
Nice, this will help a lot when it
comes to making our character jump.
7:25
In fact, let's go ahead and do that now.
7:30
In the update method on line 43,
let's expose the up and
7:33
the space properties
from the this.keys field.
7:38
At the end of the word right,
press comma and then write up,
7:43
add another comma, and then write space.
7:48
This is so
that when the user presses either up or
7:51
space on the keyboard,
we want the player to jump.
7:54
Next, below our else statement on line 51,
7:58
press Enter a few times and
write if, add parentheses.
8:01
And inside those parentheses,
add another parentheses, and
8:05
then write up.isDown, add a pipe,
and then write space.isDown.
8:10
And then outside of our yellow
parentheses, add double ampersands and
8:16
write this.#player.body.onFloor and
add parentheses.
8:23
Then add curly braces and hit Enter.
8:29
What line 53 is doing is checking if
the up or space key is pressed and
8:32
checking if the player
is touching the floor.
8:37
Now, let's add some logic for
when this happens.
8:40
On line 54,
type this.#player.setVelocityY,
8:43
add parentheses, and inside those
parentheses, add a value of -700.
8:48
This means the character will be pushed
up by 700 pixels, but then they'll
8:56
be pushed down by our gravity, and
this simulates a jumping effect.
9:01
Cool, let's see if this
works in the browser.
9:05
If we press and hold onto the right key,
then press space,
9:10
you can see the player jumps so they go
up into the air and then comes back down.
9:13
If we press and hold onto the left key and
then press jump,
9:18
we can also see that the player jumps and
then comes back down.
9:22
But you would have noticed that
when the player is jumping and
9:27
a direction is being pressed,
the running animation keeps playing.
9:30
Ideally, we don't want the player to
be running while they're in the air.
9:34
Let's fix this.
9:38
We'll need to create a new
animation with that frame, and
9:40
then only show that frame when the player
is jumping and moving in a direction.
9:43
If we go to
the spritesheet_players.xml file,
9:49
you'll see that on line 7,
there's a frame called alienBeige_jump.
9:53
This is the frame we want to
use when the player is jumping.
9:59
Let's change our code so it's using
that frame whenever the player jumps.
10:02
At the end of line 39, press Enter and
then write this.#player.anims.create,
10:07
add parentheses and
add curly braces, then hit Enter.
10:14
Then on line 41, write key : jumping,
10:19
add a comma, and then hit Enter.
10:23
And on line 42, write frames,
10:26
add square brackets,
then add curly braces,
10:30
then write key : player, add a comma,
10:35
then write frame : alienBeige_jump.png.
10:39
Cool, now let's scroll down
to our update method and
10:44
create a new variable below our keys.
10:48
At the end of line 47, hit Enter, and
10:50
write const movementAnim =
this.#player.body.onFloor.
10:55
Then add parentheses, then add
a question mark, then add running,
11:04
and then add a colon, and
then after the colon, add jumping.
11:09
This is a ternary that checks if
the character is on the floor.
11:14
And if they are,
then return the string running, and
11:18
if they're not on the floor,
then return the string jumping.
11:21
This is going to determine what
animation should be playing.
11:25
Next, on line 53, change the value of
running to our movementAnim variable.
11:30
Sweet, let's see if this has worked.
11:36
If we press and hold the right key and
then press space or up to jump,
11:40
you'll see that the jumping animation in
played and not the running animation.
11:44
We can do the same going
in the other direction.
11:49
If we press and hold the left key,
then press up or space to jump,
11:52
you'll see again that the jumping
animation is being played.
11:56
Nice, now as much as I
like this gray background,
11:59
I think it's time to give our game a few
more interesting objects to interact with.
12:03
So in the next video,
12:08
we're going to learn how to design
a level using a program called Tiled.
12:10
You need to sign up for Treehouse in order to download course files.
Sign upYou need to sign up for Treehouse in order to set up Workspace
Sign up